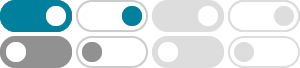
javascript - Reverse array with for loops - Stack Overflow
Jun 23, 2018 · This is similar code to reverse an Array. function reverseArray(arr) { let newArr = []; for (i = arr.length - 1; i >= 0; i--) { newArr.push(arr[i]); } return newArr; } console.log(reverseArray(["A", "B", "C", "D", "E", "F"])); //output: ["F","E","D","C","B","A"]
What is the most efficient way to reverse an array in JavaScript?
Just start copying array from the backside using a for loop and return that new array. This is efficient and has O(n) time complexity.
Reverse an Array in JavaScript - GeeksforGeeks
Jan 20, 2025 · JavaScript provides a built-in array method called reverse () that reverses the elements of the array in place. This method mutates the original array and returns the reversed array. The reverse () method directly modifies the original array by reversing its elements. 2. Using For Loop.
Reverse an array in JavaScript without mutating the original array
Aug 22, 2023 · Array.prototype.toReversed() is the new ES2023 method for returning a reversed array, without modifying the original one. const items = [1, 2, 3]; console.log(items); // [1, 2, 3] const reversedItems = items.toReversed(); console.log('reversedItems', reversedItems); // [3, 2, 1] console.log('items', items); // [1, 2, 3]
Loop through an array backward in JavaScript | Techie Delight
Apr 19, 2024 · There are several methods to loop through the array in JavaScript in the reverse direction: 1. Using reverse for-loop. The standard approach is to loop backward using a for-loop starting from the end of the array towards the beginning of the array. 2. Using Array.prototype.reverse() function.
How to Reverse an Array in JavaScript – JS .reverse() Function
Nov 29, 2022 · How to Reverse an Array Using a for Loop. Using a for loop (or any other type of loop), we can loop through an array from the last time to the first item, and push those values to a new array which becomes the reversed version. Here's how: reversedArray.push(valueAtIndex) } .
JavaScript Reverse Array – Tutorial With Example JS Code
Jan 18, 2021 · How to Reverse an Array in JavaScript Without the Reverse Method. Sometimes a job interview will challenge you to reverse an array without the reverse method. No problem! You can use the combination of a for loop and an array push method like in the example below:
How to Reverse an Array in JavaScript | TheDevSpace Community
This tutorial discusses four different ways you can reverse an array in JavaScript, including the reverse() method, for loops, recursions, and the map() method.
JavaScript: Reverse an array using different methods - Flexiple
Mar 11, 2022 · In this article, we look at different methods to reverse the array elements using JavaScript. As the name suggests, this method reverses the order of array elements by modifying the existing array. Syntax: Example: console.log(arr); //Output: [ 4, 3, 2, 1 ] for (let i = arr.length - 1; i >= 0; i--) { arr1.push(arr[i]); console.log(arr1);
Reverse an Array in JavaScript - Stack Abuse
Mar 6, 2023 · In this guide, we'll take a look at how to reverse an array or list in JavaScript, using the reverse() method, functional programming and a manual for loop - both in-place and out-of-place.
- Some results have been removed