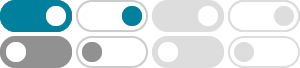
javascript - Functions that return a function: what is the difference ...
Imagine the function as a type, like an int. You can return ints in a function. You can return functions too, they are object of type "function". Now the syntax problem: because functions returns values, how can you return a function and not it's returning value? by omitting brackets! Because without brackets, the function won't be executed! So:
What does "return" do in Javascript? - Stack Overflow
Jun 10, 2017 · When a return is encountered in a function. It will return to the point immediately after the code that called the function. If nothing is specified after the return statement, that is all that happens. However if a literal is returned like 2 or 3,etc, a literal is returned. You can also return a Boolean "true/false ", a string, or a variable.
How to return values in javascript - Stack Overflow
May 4, 2011 · JavaScript passes a value from a function back to the code that called it by using the return statement. The value to be returned is specified in the return keyword. Share
Returning functions from functions in JavaScript - Stack Overflow
Dec 21, 2016 · Your own question title is basically the answer: you've written the createDrinkOrder() function as something that returns another function. If you make a function but never call it, the function won't do anything. You don't need a variable involved as in your serveOnePassenger() function. You could create the function and call it with one ...
What does "return function () { ... }" do in JavaScript?
Aug 4, 2011 · This code creates a function generator. The first function (whose reference is stored in f) accepts a reference to another function (a). Then f creates a function that closes over the parameter a and returns a reference to the new function that will …
How to use a return value in another function in Javascript?
Jul 12, 2017 · Call the function and save the return value of that very call. ... Javascript to return a function, but ...
Return value from nested function in Javascript - Stack Overflow
Nov 3, 2015 · function mainFunction() { function subFunction() { var str = "foo"; return str; } return subFunction; } var test = mainFunction(); alert( test() ); for your actual code. The return should be outside, in the main function. The callback is called somewhere inside the getLocations method and hence its return value is not recieved inside your main ...
Javascript: return function with predefined arguments
Jun 7, 2011 · Javascript: return function with predefined arguments. Ask Question Asked 16 years, 1 month ago. Modified ...
javascript - How does returning a function inside a function work ...
Aug 26, 2018 · In JavaScript functions are known as "First-class function" this means you can return / pass functions as any other type like (object / numbers). You can find more about this here . You can break this one into variables to make it more clear such as:
assign function return value to some variable using javascript
Oct 8, 2018 · Your doSomething function is being exectued, the AJAX request is being made but it happens asynchronously; so the remainder of doSomething is executed and the value of status is undefined when it is returned. Effectively, your code works as follows: function doSomething(someargums) { return status; } var response = doSomething();