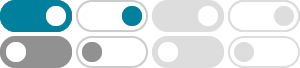
Python reversing a string using recursion - Stack Overflow
I want to use recursion to reverse a string in python so it displays the characters backwards (i.e "Hello" will become "olleh"/"o l l e h". I wrote one that does it iteratively: def Reverse( s ): result = "" n = 0 start = 0 while ( s[n:] != "" ): while ( s[n:] != "" and s[n] != ' ' ): n = n + 1 result = s[ start: n ] + " " + result start = n ...
Print reverse of a string using recursion - GeeksforGeeks
Mar 6, 2025 · Given a string, the task is to print the given string in reverse order using recursion. Examples: Input: s = “Geeks for Geeks” Output: “skeeG rof skeeG“ Explanation: After reversing the input string we get “skeeG rof skeeG“. Input: s = “Reverse a string Using Recursion” Output: “noisruceR gnisU gnirts a esreveR“
How to reverse a String in Python - GeeksforGeeks
Nov 21, 2024 · Using reversed () and join () Python provides a built-in function called reversed (), which can be used to reverse the characters in a string. This approach is very useful if we prefer to use built-in methods.
Reverse Strings in Python: reversed (), Slicing, and More
Python provides two straightforward ways to reverse strings. Since strings are sequences, they’re indexable, sliceable, and iterable. These features allow you to use slicing to directly generate a copy of a given string in reverse order.
Reverse a String Using Recursion in Python - Online Tutorials …
Mar 12, 2021 · Learn how to reverse a string using recursion in Python with this step-by-step guide and example.
python - How to reverse a string using recursion? - Stack Overflow
In python, you can reverse strings in one simple line, and avoid recursive entirely, unless it is some assignment with a requirement. So do: s[::-1], where s is the variable name of the string to be reversed.
Reverse a String Using Recursion in Python – allinpython.com
In this post, we learn how to write a program to Reverse a String Using Recursion in Python with a detailed explanation and algorithm.
Reverse a String in Python - Sanfoundry
In this approach, we use recursion to reverse a string by repeatedly swapping the first and last characters. Here is source code of the Python Program to reverse a string using recursion. The program output is also shown below. if len(string) == 0: return string else: return reverse (string[1:]) + string[0] . 1. User must enter a string. 2.
Python String Reversal
String reversal is a common programming task that can be approached in multiple ways in Python. This comprehensive guide will explore various methods to reverse a string, discussing their pros, cons, and performance characteristics.
5 Best Ways to Reverse a String in Python Using Recursion
Mar 7, 2024 · This article demonstrates five recursive methods to achieve this in Python. One straightforward approach to reverse a string using recursion involves creating a function that concatenates the last character of the string with a recursive call that excludes this character.
- Some results have been removed