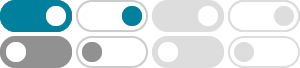
Python reversing a string using recursion - Stack Overflow
I want to use recursion to reverse a string in python so it displays the characters backwards (i.e "Hello" will become "olleh"/"o l l e h". I wrote one that does it iteratively: result = "" n = 0. start = 0. while ( s[n:] != "" ): while ( s[n:] != "" and s[n] != ' ' ): n = n + 1. result = s[ start: n ] + " " + result. start = n. return result.
Print reverse of a string using recursion - GeeksforGeeks
Mar 6, 2025 · Given a string, the task is to print the given string in reverse order using recursion. Examples: Input: s = “Geeks for Geeks” Output: “ skeeG rof skeeG “ Explanation: After reversing the input string we get “ skeeG rof skeeG “. Input: s = “Reverse a string Using Recursion” Output: “ noisruceR gnisU gnirts a esreveR “
Reverse a String Using Recursion in Python - Online Tutorials …
Mar 12, 2021 · Learn how to reverse a string using recursion in Python with this step-by-step guide and example.
python - How to reverse a string using recursion? - Stack Overflow
In python, you can reverse strings in one simple line, and avoid recursive entirely, unless it is some assignment with a requirement. So do: s[::-1], where s is the variable name of the string to be reversed.
Reverse a String Using Recursion in Python – allinpython.com
These three steps help you to reverse a string using recursion. Source Code def reverse_str(my_str): if len(my_str) == 0: return my_str else: return reverse_str(my_str[1:]) + my_str[0] my_string = input('Enter your string:') print(f"Given String: {my_string}") print(f"reversed String: {reverse_str(my_string)}")
5 Best Ways to Reverse a String in Python Using Recursion
Mar 7, 2024 · This article demonstrates five recursive methods to achieve this in Python. Method 1: Basic Recursion. One straightforward approach to reverse a string using recursion involves creating a function that concatenates the last character of the string with a recursive call that excludes this character.
Python Reverse String – String Reversal in Python Explained …
Nov 10, 2021 · how to recursively reverse a string – go on until you have an empty string or have only one character left (this would work fine too, as one character reversed is itself), how to use <string>[::-1] to get a reversed copy of <string> , and
Reverse a String in Python - Sanfoundry
Here is a Python program to reverse a string using loops, recursion and slice function with detaield explanation and examples.
How to reverse a String in Python (5 Methods)
Jan 24, 2024 · Reversing a string is a common operation in programming, and Python provides multiple ways to achieve this task. This blog post explores five distinct methods to reverse a string, each with its unique approach.
Python String Reversal
String reversal is a common programming task that can be approached in multiple ways in Python. This comprehensive guide will explore various methods to reverse a string, discussing their pros, cons, and performance characteristics.
- Some results have been removed