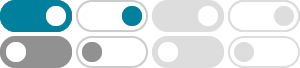
Python Program to Display Fibonacci Sequence Using Recursion
A recursive function recur_fibo() is used to calculate the nth term of the sequence. We use a for loop to iterate and calculate each term recursively. Also Read:
Print the Fibonacci sequence – Python | GeeksforGeeks
Mar 22, 2025 · Using Recursion . This approach uses recursion to calculate the Fibonacci sequence. The base cases handle inputs of 0 and 1 (returning 0 and 1 respectively). For values greater than 1, it recursively calls the function to sum the previous two Fibonacci numbers.
Fibonacci Series in Python using Recursion - Python Examples
Learn to generate the Fibonacci series in Python using recursion. Explore two methods, comparing brute force and optimized recursive approaches.
A Python Guide to the Fibonacci Sequence
In this step-by-step tutorial, you'll explore the Fibonacci sequence in Python, which serves as an invaluable springboard into the world of recursion, and learn how to optimize recursive algorithms in the process.
python - How to create a recursive function to calculate Fibonacci ...
Jan 10, 2014 · Your function is already recursive. You simply need to pass in a number other than 0, 1, or 5 to see the effect: >>> def fib(n): ... if n == 0: ... return 0 ... elif n ==1: ... return 1 ... else: ... return fib (n-1) + fib (n-2) ...
Fibonacci in python, recursively into a list - Stack Overflow
Jun 14, 2016 · In Python 3 you can do an efficient recursive implementation using lru_cache, which caches recently computed results of a function: if N <= 1: return 1. return fib(N - 1) + fib(N - 2) This also works without the cacheing, but computational complexity is …
Python Program To Print Fibonacci Series Using Recursion
In this code, we define a recursive function called fibonacci(). This function takes an integer n as input and returns the nth term of the Fibonacci series. If n is 0 or 1, we simply return n. Otherwise, we calculate the nth term by recursively calling the fibonacci function with arguments n-1 and n-2, and adding the results.
Python Fibonacci Recursive Solution - DevRescue
Jan 16, 2024 · In this implementation, the function fibonacci_verbose takes an integer n and returns the nth number in the Fibonacci sequence. The base case handles the first two numbers of the sequence, 0 and 1, corresponding to n == 0 and n == 1 , respectively.
Unraveling Fibonacci: A Recursive Journey in Python - Computer …
Dec 13, 2023 · Below is a Python program that prints Fibonacci sequences up to 'n' numbers using recursion: def fibonacci (n): if n <= 0: return "Please enter a positive integer." elif n == 1: return [1] elif n == 2: return [1, 1] else: fib_sequence = fibonacci (n - 1) fib_sequence.append (fib_sequence [-1] + fib_sequence [-2]) return fib_sequence.
Recursive Fibonacci in Python (for Beginners) - Medium
Jan 6, 2025 · It introduced me to Fibonacci sequence and how to use Memoization to speed up the calculation in Python. However, I found myself a bit puzzled by the code execution sequence and how caching...