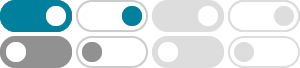
C Program for Binary Search - GeeksforGeeks
Aug 12, 2024 · In this article, we will understand the binary search algorithm and how to implement binary search programs in C. We will see both iterative and recursive approaches and how binary search can reduce the time complexity of …
C Program for Binary Search - CodesCracker
Binary Search Program in C using Recursion. This is the last program on binary search. This program used a recursive function to find the number from the given array using the binary search technique. Let's take a look at the program:
Binary Search Program in C - C Language Basics
Sep 18, 2014 · Binary search program in c using function and without using function is given below with the output. Binary search is a divide and conquer search algorithm used primarily to find out the position of a specified value within an array.
Binary Search Program in C using Recursive and Non-Recursive Methods
/* Binary search program in C using both recursive and non recursive functions */ #include <stdio.h> #define MAX_LEN 10 /* Non-Recursive function*/ void b_search_nonrecursive(int l[],int num,int ele) { int l1,i,j, flag = 0; l1 = 0; i = num-1; while(l1 <= i) { j = (l1+i)/2; if( l[j] == ele) { printf("\nThe element %d is present at position %d in ...
Binary Search (With Code) - Programiz
Binary Search is a searching algorithm for finding an element's position in a sorted array. In this tutorial, you will understand the working of binary search with working code in C, C++, Java, and Python.
Binary Search in C - Code Revise
Here you will learn Binary Search algorithm and the program code of Binary Search in C language by using 4 different ways like with function, with recursion, with arrays and without functions.
C Program To Perform Binary Search Using Recursion
Jan 20, 2022 · Binary Search is an efficient method to find the target value from the given ordered items, In Binary Search the key given value is compared with the middle value, of an array, when the key value is less than or greater than the given array, the algorithm knows from where to search the given value.
Binary Search: Recursive and Iterative in C Program
Learn how to implement binary search using both recursive and iterative methods in C programming. Step-by-step guide with examples. Discover how to perform binary search in C with recursive and iterative techniques.
Binary Search Algorithm in C - Sanfoundry
This is a C Program to search an element in an Array using Binary Search Algorithm using recursion. Problem Description We have to create a C Program which inputs a sorted array and tells whether the key searched is present in array or …
Write a C program that uses non recursive function to search for …
Write C programs that use both recursive and non-recursive functions To find the factorial of a given integer. To find the GCD (greatest common divisor) of two given integers.