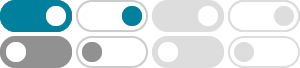
Tower of Hanoi: Recursive Algorithm - Stack Overflow
Feb 7, 2016 · After reading all these explanations I thought I'd weigh in with the method my professor used to explain the Towers of Hanoi recursive solution. Here is the algorithm again with n representing the number of rings, and A, B, C representing the pegs. The first parameter of the function is the number of rings, second parameter represents the ...
What is recursion and when should I use it? - Stack Overflow
A recursive function is a function that contains a call to itself. A recursive struct is a struct that contains an instance of itself. You can combine the two as a recursive class. The key part of a recursive item is that it contains an instance/call of itself. Consider two mirrors facing each other. We've seen the neat infinity effect they make.
Algorithm to return all combinations of k elements from n
Sep 24, 2008 · Simple recursive algorithm in Haskell. import Data.List combinations 0 lst = [[]] combinations n lst = do (x:xs) <- tails lst rest <- combinations (n-1) xs return $ x : rest We first define the special case, i.e. selecting zero elements. It produces a single result, which is an empty list (i.e. a list that contains an empty list).
algorithm - Pseudocode recursive function - Stack Overflow
Oct 15, 2013 · 1) Explain why for any integer a>= 1 algorithm MILK(a) terminates. I think for this one because of the n-1, the possibility for m becomes smaller and smaller for the input into the recursive function MILK(b);, eventually reaching 1 which satisfies condition a = 1; therefore eating a cookie and so terminating the algorithm.
Can every recursion be converted into iteration? - Stack Overflow
May 31, 2009 · Yes. A simple formal proof is to show that both µ recursion and a non-recursive calculus such as GOTO are both Turing complete. Since all Turing complete calculi are strictly equivalent in their expressive power, all recursive functions can be implemented by the non-recursive Turing-complete calculus.
Real-world examples of recursion - Stack Overflow
Sep 20, 2008 · Recursion is appropriate whenever a problem can be solved by dividing it into sub-problems, that can use the same algorithm for solving them. Algorithms on trees and sorted lists are a natural fit. Many problems in computational geometry (and 3D games) can be solved recursively using binary space partitioning (BSP) trees, fat subdivisions , or ...
Is Dijkstra's algorithm dynamic programming? - Stack Overflow
May 6, 2024 · Recursion gives us a stack. But we don't need a stack here. We need a priority queue. The efficient way to implement Dijkstra's algorithm uses a heap (stl priority_queue in c++). but I have also read that by definition dynamic programming is an algorithm with a recursive function and "memory" of things already calculated.
java - Recursive Algorithm for 2D maze? - Stack Overflow
Nov 25, 2013 · All these characters of the maze is stored in 2D array. The program must find the path from start 'S' to goal 'G'. For this, a boolean method called 'solve(int row, int col) is uses and is initialized with row and column index of 'S'. The algorithm must be recursive. It should return true if its able to find the path to 'G' and false other wise.
Understanding Recursion to generate permutations - Stack Overflow
Under each loop we are recursively calling with LpCnt + 1. 4.1 When index is 1 then 2 recursive calls. 4.2 When index is 2 then 1 recursive calls. So from point 2 to 4.2 total calls are 5 for each loop and total is 15 calls + main entry call = 16. Each …
Determining complexity for recursive functions (Big O notation)
Nov 20, 2012 · One of the best ways I find for approximating the complexity of the recursive algorithm is drawing the recursion tree. Once you have the recursive tree: Complexity = length of tree from root node to leaf node * number of leaf nodes The first function will have length of n and number of leaf node 1 so complexity will be n*1 = n