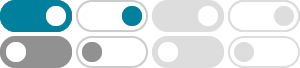
JavaScript Program for Quick Sort - GeeksforGeeks
Sep 20, 2023 · Quick sort is one of the sorting algorithms that works on the idea of divide and conquer. It takes an element as a pivot and partitions the given array around that pivot by placing it in the correct position in the sorted array.
How To Write Quick Sort Algorithm With JavaScript
Mar 15, 2023 · Quick sort is a widely used sorting algorithm that efficiently sorts an array of elements by dividing it into smaller subarrays based on a chosen pivot element. In this article, we will walk through how to write a quick sort algorithm using JavaScript and explore the key concepts behind the algorithm. What Is the Quick Sort Algorithm?
How to implement a stable QuickSort algorithm in JavaScript
Mar 3, 2011 · function quickSort(arr) { if (arr.length < 2) { return arr; } const pivot = arr[Math.floor(Math.random() * arr.length)]; let left = []; let right = []; let equal = []; for (let val of …
How To Write Quick Sort Algorithm With JavaScript: An In-Depth …
Aug 28, 2024 · In this comprehensive 3,000+ word guide, you will gain an in-depth understanding of quicksort in JavaScript, including: How quicksort provides blazing speed by exploiting divide-and-conquer ; Step-by-step walkthrough of implementation from a coder‘s perspective; Optimization techniques used in real-world quicksort variants; Comparative ...
QuickSort Algorithm in JavaScript - Guru99
Mar 9, 2024 · What is Quick sort? Quick sort follows Divide and Conquer algorithm. It is dividing elements in to smaller parts based on some condition and performing the sort operations on those divided smaller parts. Hence, it works well for large datasets. So, here are the steps how Quick sort works in simple words.
Quick Sort in JavaScript - Doable Danny
We'll cover: what is Quick Sort?; Quick Sort logic; Quick Sort steps; Implement Quick Sort in JavaScript; What is the time complexity of Quick Sort?; Advantages and disadvantages of Quick Sort; Quick Sort vs Merge Sort
Quicksort in JavaScript - Stack Abuse
Sep 21, 2023 · In this article, we'll take a look at how to implement the Quicksort algorithm. We'll go through the recursive and iterative approach, and take a look at the efficiency of Quicksort.
Implement Quick Sort in JavaScript - Online Tutorials Library
Learn how to implement the Quick Sort algorithm in JavaScript with a step-by-step guide and code examples.
Understanding Quick Sort via JavaScript - DigitalOcean
Feb 14, 2020 · Using JavaScript, learn one of the most popular and professional sorting algorithms: quick sort.
Quick Sort Algorithm With JavaScript - DEV Community
Jan 19, 2022 · This article's aim is to explain in detail one of these sorting algorithms. It is the Quick Sort. Table of Content What is Quick Sort; Terminologies; How Quick Sort Works; Technically, Quick Sort follows the below steps; Analysis of Quick Sort's Time Complexity; How best to pick a pivot; Implementation of Quick Sort; Prerequisite; Method 1 ...
- Some results have been removed