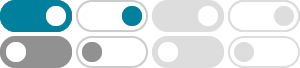
Implementation of Queue in Javascript - GeeksforGeeks
Feb 11, 2025 · In this implementation, we use a JavaScript array to simulate a queue, with push () to add elements to the end and shift () to remove elements from the front. class Queue { constructor() { this.items = []; } enqueue(element) { this.items.push(element); } dequeue() { return …
How do you implement a Stack and a Queue in JavaScript?
function newQueue() { return { headIdx: 0, tailIdx: 0, elts: {}, enqueue: (elt) => queue.elts[queue.tailIdx++] = elt, dequeue: => { if (queue.headIdx == queue.tailIdx) { throw new Error("Queue is empty"); } return queue.elts[queue.headIdx++]; }, size: => queue.tailIdx - queue.headIdx, isEmpty: => queue.tailIdx == queue.headIdx }; }
JavaScript Queue
A queue works based on the first-in, first-out (FIFO) principle, which is different from a stack, which works based on the last-in, first-out (LIFO) principle. A queue has two main operations: Insert a new element at the end of the queue, which is called enqueue. Remove an element from the front of the queue, which is called dequeue.
Implement enqueue and dequeue using only two stacks in JavaScript ...
Jan 13, 2022 · In this article, we will implement queue’s operations (enqueue and dequeue) using only two stacks (in the form of plain arrays) in JavaScript. Before directly jumping into understanding the problem statement let us understand briefly what exactly a stack is and also what exactly a queue is.
How do I store javascript functions in a queue for them to be …
I have created a Queue class in javascript and I would like to store functions as data in a queue. That way I can build up requests (function calls) and respond to them when I need to (actually executing the function).
How to Implement a Queue in JavaScript - Dmitri Pavlutin Blog
Calling queue.enqueue(7) method enqueues the item 7 into the queue. queue.dequeue() dequeues a head item from the queue, while queue.peek() just peeks the item at the head. Finally, queue.length shows how many items are still in the queue.
JavaScript Program to Implement a Queue (With Example)
The enqueue() method adds elements to the queue. The dequeue() method removes the first element from the queue. The peek() method returns the first element from the queue.
Queue In JavaScript - Medium
Jul 8, 2020 · A standard queue has three methods: peek, enqueue, and dequeue. Our peek method will view the node that is in the front of our queue. Since in a queue we can only add items to the back, our...
JavaScript queue data structure implementation tutorial
May 14, 2021 · The enqueue() method takes an element as its parameter and puts it in the tail index. Once the new element is stored, you need to increment the tail pointer by one: enqueue ( element ) { this . elements [ this . tail ] = element ; this . tail ++ ; }
Understanding Queues in JavaScript: From Concept to Code
We learned how queues operate on the First-In, First-Out (FIFO) principle and how to implement them using JavaScript arrays. The lesson covered enqueue and dequeue operations, the isEmpty method, time and space complexity analysis for queue operations, and discussed real-world scenarios where queues are an effective data structure.
- Some results have been removed