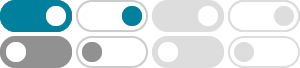
python - How can I catch multiple exceptions in one line? (in the ...
As of Python 3.11 you can take advantage of the except* clause that is used to handle multiple exceptions. PEP-654 introduced a new standard exception type called ExceptionGroup that corresponds to a group of exceptions that are being propagated together.
python - One try block with multiple excepts - Stack Overflow
In Python, is it possible to have multiple except statements for one try statement? Such as: try: #something1 #something2 except ExceptionType1: #return xyz except ExceptionType2: #return abc
python - Multiple try codes in one block - Stack Overflow
If you don't want to chain (a huge number of) try-except clauses, you may try your codes in a loop and break upon 1st success. Example with codes which can be put into functions: for code in ( lambda: a / b, lambda: a / (b + 1), lambda: a / (b + 2), ): try: print(code()) except Exception as ev: continue break else: print("it failed: %s" % ev)
How to Catch Multiple Exceptions in Python
In this how-to tutorial, you'll learn different ways of catching multiple Python exceptions. You'll review the standard way of using a tuple in the except clause, but also expand your knowledge by exploring some other techniques, such as suppressing exceptions and using exception groups.
Multiple Exception Handling in Python - GeeksforGeeks
Jun 12, 2019 · In this article, we will learn about how we can have multiple exceptions in a single line. We use this method to make code more readable and less complex. Also, it will help us follow the DRY (Don't repeat code) code method.
Python Try Except: Handling Errors Like a Pro
Use multiple except blocks for specific exceptions and implement finally blocks for critical cleanup. Keep code concise and specify exception types for clarity. Avoid bare except clauses and overuse of try-except blocks.
Python Try Except: Examples And Best Practices
Sep 24, 2024 · In this article, you will learn how to handle errors in Python by using the Python try and except keywords. It will also teach you how to create custom exceptions, which can be used to define your own specific error messages.
How to Catch Multiple Exceptions in Python? - Python Guides
Mar 20, 2025 · Learn how to catch multiple exceptions in Python using try-except blocks. Handle errors efficiently with best practices and examples. Read our guide now!
Python Nested Try Except (A Comprehensive Guide) - Python …
Multi-level Error Recovery: By nesting try-except blocks, you can handle errors at different levels of code execution and perform appropriate actions based on the specific error encountered. Let’s look at a couple of examples to understand how nested try-except blocks work in practice. # Outer try block. # Code that might raise an exception. try:
How to catch multiple exceptions in Python? [SOLVED]
Nov 28, 2023 · Catch multiple exceptions inside single except block. You can catch multiple exceptions in one except block in Python using parentheses to specify the exception types. Here is an example syntax: try: # code that might raise an exception except (ExceptionType1, ExceptionType2, ExceptionType3): # code to handle the exception
- Some results have been removed