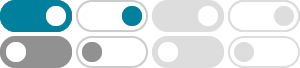
Python program to find the factorial of a number using recursion
Jan 31, 2023 · In this article, we are going to calculate the factorial of a number using recursion. Examples: Output: 120. Input: 6. Output: 720. Implementation: If fact (5) is called, it will call fact (4), fact (3), fact (2) and fact (1). So it means keeps calling itself by …
Factorial of a Number – Python - GeeksforGeeks
Apr 8, 2025 · This Python program uses a recursive function to calculate the factorial of a given number. The factorial is computed by multiplying the number with the factorial of its preceding number.
Python Program to Find the Factorial of a Number
# Python program to find the factorial of a number provided by the user # using recursion def factorial(x): """This is a recursive function to find the factorial of an integer""" if x == 1 or x == 0:
Python program that uses recursion to find the factorial of a …
Jan 13, 2023 · In this blog post, we’ll explore a Python program that uses recursion to find the factorial of a given number. The post will provide a comprehensive explanation along with a step-by-step guide and example outputs.
Python Program For Factorial (3 Methods With Code)
A factorial program in Python with recursion is a program that calculates the factorial of a number using a recursive approach. It calls itself repeatedly until it reaches a base case (typically when the number is 0), and then returns the factorial.
Function for factorial in Python - Stack Overflow
Jan 6, 2022 · How do I go about computing a factorial of an integer in Python? The easiest way is to use math.factorial (available in Python 2.6 and above): If you want/have to write it yourself, you can use an iterative approach: fact = 1. for num in range(2, n + 1): fact *= num. return fact. or a recursive approach: if n < 2: return 1. else:
Factorial of a Number in Python using Recursion - Know Program
# Python program to find the factorial of a number using recursion def recur_factorial(n): #user-defined function if n == 1: return n else: return n*recur_factorial(n-1) # take input
Program to find the factorial of a number using Recursion
Nov 22, 2024 · def factorial (n): # Base case: factorial of 0 or 1 is 1 if n == 0 or n == 1: return 1 else: # Recursive case: n * factorial of (n - 1) return n * factorial (n - 1) def main (): try: # Input the number number = int (input ("Enter a number: ")) # Check for negative numbers if number < 0: print ("Factorial cannot be calculated for negative ...
Write a Python Program to Find Factorial of Number Using Recursion
In this tutorial, we will learn how to find the factorial of a number using recursion in Python programming language. Factorial is a mathematical function denoted by an exclamation mark (!). The factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n.
Python Program to Find Factorial of Number Using Recursion
In this program, you'll learn to find the factorial of a number using recursive function.
- Some results have been removed