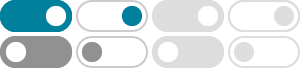
Function for factorial in Python - Stack Overflow
Jan 6, 2022 · def factorial(n): return 1 if n == 0 else n * factorial(n-1) One line lambda function approach: (although it is not recommended to assign lambda functions directly to a name, as it is considered a bad practice and may bring inconsistency to your code. It's always good to know. See PEP8.) factorial = lambda n: 1 if n == 0 else n * factorial(n-1)
python - recursive factorial function - Stack Overflow
def factorial(n): while n >= 1: return n * factorial(n - 1) return 1 Although the option that TrebledJ wrote in the comments about using if is better. Because while loop performs more operations (SETUP_LOOP, POP_BLOCK) than if. The function is slower. def factorial(n): if n >= 1: return n * factorial(n - 1) return 1
Factorial of a large number in python - Stack Overflow
May 1, 2013 · $ python factorial.py 81430 math.factorial took 4.06193709373 seconds 81430 factorial took 3.84716391563 seconds 81430 treefactorial took 0.344486951828 seconds so a more than 10× speedup for 100000!.
Writing a Factorial function in one line in Python
Aug 10, 2018 · You can use reduce to write a factorial function. from operator import mul # from functools import reduce # Python3 only def factorial(x): return reduce(mul, range(1, x + 1), 1) factorial(5) # 24 You can also import it from the math module which additionally raises exceptions for negative and non-integral numbers. from math import factorial
Using Reduce Function in Python To Find Factorial
Oct 2, 2014 · import functools def factorial(n): if n == 0: return 1 else: return functools.reduce(lambda x,y: x*y, range(1,n+1)) print factorial(3) Of course you can use you own multi function instead of the lambda if you prefer.
Python lambda function to calculate factorial of a number
Mar 14, 2013 · The factorial itself is almost as you'd expect it. You infer that the a is... the factorial function. b is the actual parameter. <factorial> = lambda a, b: b*a(a, b-1) if b > 0 else 1 This bit is the application of the factorial: <factorial-application> = (lambda a, b: a(a, b))(<factorial>, b) a is the factorial function itself. It takes itself ...
algorithm - Python - Sum of the factorials - Stack Overflow
Feb 25, 2015 · math.factorial(x) Initialize a sum with 0 , use a for loop and add the result of the above line to the sum: from math import factorial s=0 m=4 for k in range (1,m+1) : s=s+factorial(k) print (s)
what is wrong with my factorial code in python - Stack Overflow
Feb 12, 2015 · The performance difference becomes more apparent if you try to compute the factorial of larger numbers: python -m timeit -s "import original" "original.factorial(30)" 100000 loops, best of 3: 5.25 usec per loop vs. python -m timeit -s "import func3" "func3.factorial(30)" 100000 loops, best of 3: 3.96 usec per loop
Python: Using def in a factorial program - Stack Overflow
This will define a factorial function and a main function. The if block at the bottom will execute the main function, but only if the script is interpreted directly: ~> python3 test.py Please enter a number greater than or equal to 0: 4 4 factorial is 24 Alternatively, you can import your script into another script or an interactive session.
syntax - calculating factorial in Python - Stack Overflow
Aug 2, 2012 · what is wrong with my factorial code in python. 0. Calculating factorials with Python. 4.