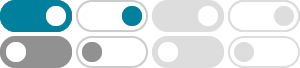
Manually raising (throwing) an exception in Python
When inside an except clause, you might want to, for example, log that a specific type of error happened, and then re-raise. The best way to do this while preserving the stack trace is to use a bare raise statement. For example: do_something_in_app_that_breaks_easily() logger.error(error) raise …
else & elif statements not working in Python - Stack Overflow
The if statement is working all fine, but when I write else or elif commands, the interpreter gives me a syntax error. I'm using Python 3.2.1 and the problem is arising in both its native interpreter and IDLE.
Python gives a "Syntax Error" on "else:" - Stack Overflow
Python throws a syntax error pointed at the last "e" of "else:", preceded by an if statement and inside a while loop. Test if certain parameters are true, if true then go to the beginning of the loop and if not true then perform certain statements and increment a value. digs = [] while len(str(num)) != 1: num = str(num) for each in num:
Python Raise an Exception - W3Schools
To throw (or raise) an exception, use the raise keyword. The raise keyword is used to raise an exception. You can define what kind of error to raise, and the text to print to the user.
8. Errors and Exceptions — Python 3.13.3 documentation
1 day ago · Even if a statement or expression is syntactically correct, it may cause an error when an attempt is made to execute it. Errors detected during execution are called exceptions and are not unconditionally fatal: you will soon learn how to handle them in Python programs.
Error Handling in Python – try, except, else, & finally Explained …
Apr 11, 2024 · Try and Except statements follow a pattern that allows you to reliably handle problems in your code. Let’s go over the pattern. The first step that happens is, the code in the try clause attempts to execute. After that, we have three possibilities: No Errors in the Try Clause. If the code in the try clause executes without any errors, the ...
Python Conditional Statements - Python Guides
Conditional statements in Python let you execute specific blocks of code only when certain conditions are met. Think of them as the decision-makers in your code. Python offers three main types of conditional statements: if statement; if-else statement; if-elif-else statement; The Simple if Statement. The if statement is the most
How to Handle Errors in Python – the try, except, else, and finally ...
Nov 1, 2022 · try: a = int(input()) b = int(input()) result = a/b print(result) except: print("We caught an error") else: print("Hurray, we don't have any errors") finally: print("I have reached the end of the line")
Built-in Exceptions — Python 3.13.3 documentation
1 day ago · In Python, all exceptions must be instances of a class that derives from BaseException. In a try statement with an except clause that mentions a particular class, that clause also handles any exception classes derived from that class (but not exception classes from which it is derived).
How to Fix Else Syntax Error in Python: Quick and Easy Guide
Dec 13, 2024 · Correcting an else syntax error often involves checking for matching if or elif statements and ensuring all lines are properly indented. By adhering to these practices, you can write clean, error-free Python code. Credit: stackoverflow.com . Common Causes. Encountering an Else Syntax Error in Python can be frustrating. But knowing the common ...