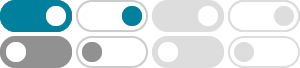
Find max or min value in an array of primitives using Java
Apr 9, 2025 · To get the minimum or maximum value from the array we can use the Collections.min() and Collections.max() methods. But as this method requires a list type of data we need to convert the array to list first using above explained “ aslist() ” function.
Finding the max/min value in an array of primitives using Java
Mar 13, 2015 · It's trivial to write a function to determine the min/max value in an array, such as: * @param chars. * @return the max value in the array of chars. */ int max = chars[0]; for (int ktr = 0; ktr < chars.length; ktr++) { if (chars[ktr] > max) { max = chars[ktr]; return max; but isn't this already done somewhere?
Program to find the minimum (or maximum) element of an array
Apr 10, 2025 · Given an array A[] of N integers and two integers X and Y (X ≤ Y), the task is to find the maximum possible value of the minimum element in an array A[] of N integers by adding X to one element and subtracting Y from another element any number of times, where X ≤ Y. Examples: Input: N= 3, A[] = {1,
Java Minimum and Maximum values in Array - Stack Overflow
Aug 30, 2013 · public static void getMinMaxByArraysMethods(int[] givenArray){ //Sum of Array in One Line long sumofArray = Arrays.stream(givenArray).sum(); //get Minimum Value in an array in One Line int minimumValue = Arrays.stream(givenArray).min().getAsInt(); //Get Maximum Value of an Array in One Line int MaxmumValue = Arrays.stream(givenArray).max ...
Finding Min/Max in an Array with Java - Baeldung
Jan 8, 2024 · In this short tutorial, we’re going to see how to find the maximum and the minimum values in an array, using Java 8’s Stream API. We’ll start by finding the minimum in an array of integers, and then we’ll find the maximum in an array of objects.
Java Array Minimum position – Finding the min element
Aug 28, 2024 · It is common in Java to encounter a method which finds in Java array, the minimum element position. By iterating through the array and comparing each element to the current minimum, we can efficiently determine the index of the smallest value.
Integer.MAX_VALUE and Integer.MIN_VALUE in Java with …
Jan 22, 2020 · Integer.MIN_VALUE is a constant in the Integer class of java.lang package that specifies that stores the minimum possible value for any integer variable in Java. The actual value of this is. Any integer variable cannot store any value below this limit. Upon doing so, the memory will overflow and the value will get positive.
Three ways to find minimum and maximum values in a Java array …
In Java you can find maximum or minimum value in a numeric array by looping through the array. Here is the code to do that.
Finding Minimum and Maximum Values in an Array: Effective …
Nov 6, 2024 · Here, we’ll cover six different methods for finding minimum and maximum values in an array int[] arr = {5, 2, 7, 4, 8, 5, 9, 6}, each with its unique advantages and use cases. 1. Using Arrays.stream() (Java 8+) This approach leverages Java Streams to find the minimum and maximum values in a concise, readable way.
Find Max and Min in an Array in Java - HowToDoInJava
Feb 21, 2023 · Learn ways to find the maximum and the minimum element from an Array in Java using Stream API, Collections, simple iterations and recursion.