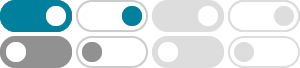
Merge Sort in Python - GeeksforGeeks
Feb 21, 2025 · Merge Sort is a comparison-based sorting algorithm that uses divide and conquer paradigm to sort the given dataset. It divides the dataset into two halves, calls itself for these two halves, and then it merges the two sorted halves.
Merge Sort – Data Structure and Algorithms Tutorials
Jan 29, 2025 · Here’s a step-by-step explanation of how merge sort works: Divide: Divide the list or array recursively into two halves until it can no more be divided. Conquer: Each subarray is sorted individually using the merge sort algorithm. Merge: The sorted subarrays are merged back together in sorted order. The process continues until all elements ...
Divide and Conquer in Python - GeeksforGeeks
May 6, 2024 · One well-known example of a divide-and-conquer algorithm is merge sort. An overview of its general operation for sorting a list of numbers is provided here: Divide: Return the list itself since it has already been sorted if there is only one element in the list.
Merge Sort (With Code in Python/C++/Java/C) - Programiz
Merge Sort is one of the most popular sorting algorithms that is based on the principle of Divide and Conquer Algorithm. Here, a problem is divided into multiple sub-problems. Each sub-problem is solved individually. Finally, sub-problems are combined to form the final solution.
Implementing the Merge Sort Algorithm in Python - Codecademy
Mar 24, 2025 · In this tutorial, we will explore how to implement merge sort in Python, a powerful sorting algorithm that uses a divide-and-conquer approach. We’ll learn how it works and how to implement it in Python and discuss its real-world applications.
Merge Sort in Python (with code) - FavTutor
Jul 6, 2023 · Algorithm for Merge Sort. The divide and conquer approach to sorting n numbers using merge sort consists of separating the array T into two parts where the sizes are almost the same. These two parts are sorted by recursive calls and then merged with the solution of each part while preserving the order.
Divide and Conquer: Merge Sort | in Python - Medium
Feb 16, 2023 · Merge sort is a divide-and-conquer algorithm that works by recursively breaking down a list into smaller sublists, sorting those sublists, and then merging the sorted sublists back together to...
Merge Sort Algorithm - Python Examples
Merge sort is a divide-and-conquer sorting algorithm that divides the input array into two halves, recursively sorts each half, and then merges the sorted halves to produce the sorted array. It has a time complexity of O (n log n) and is efficient for large data sets. Consider an array with the following elements:
Merge Sort Algorithm in Python - Alps Academy
Learn how to implement the merge sort algorithm in Python with a step-by-step guide, complete code, and a video tutorial. Understand the concept of divide and conquer algorithms and master the art of efficient sorting in Python.
Merge Sort Python Tutorial - An Efficient Way Of Sorting
Dec 1, 2018 · Merge sort based on Divide and Conquer approach. That means it divides the array into two parts, those sub-arrays will be divided into other two equal parts. We will divide the array in this manner until we get single element in each part because single element is already sorted.