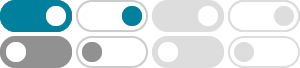
Evaluating a string as a mathematical expression in JavaScript
How do I parse and evaluate a mathematical expression in a string (e.g. '1+1') without invoking eval(string) to yield its numerical value? With that example, I want the function to accept '1+1' and return 2.
How can I convert a string into a math operator in javascript
May 11, 2016 · One way would be to simply loop over the string and check hasOwnProperty on your math_it_up object for each element; if it's true, call it with the preceding and succeeding indices in the array as the arguments to the function.
Check if string is a mathematical expression in JavaScript
Feb 27, 2018 · Here's a regex that can do what I think you're looking for: https://regex101.com/r/w74GSk/4. It matches a number, optionally negative, with an optional decimal number followed by zero or more operator/number pairs. It also allows for whitespace between numbers and operators. console.log("%s is valid? %s", s, re.test(s));
Evaluate String as Mathematical Expression in JavaScript
Nov 21, 2020 · Learn how to evaluate a string as a mathematical expression in JavaScript with this comprehensive guide.
A Simple Calculator Implemented With Javascript That Evaluates String …
Oct 23, 2021 · In this article, you will learn how to write a calculator using Javascript that can evaluate elementary arithmetic expressions. For example 2 * (2+3 / (3 -8)).
Javascript to Evaluate a String as Mathematical Expression
Introduction: In this article I am going to explain how to evaluate/ calculate a string expression as a mathematical expression using jquery/Javascript’s eval () function.
Strings and Basic Mathematical Operators in JavaScript
Oct 10, 2020 · Let us start with a brief on strings in JavaScript. Then in the next section, we will see the relationship of strings with the most common mathematical operators.
Any clean way to convert an arithmetic operator inside a string to …
Apr 2, 2022 · When you build a parser, it will recognize which parts of the input string denote operators and which don't. Once the operator tokens are isolated, you can use a simple object to translate them into what they are supposed to do. { "+": (a, b) => a + b, "-": (a, b) => a - b, "*": (a, b) => a * b, /* and so on and so forth... */ }
javascript - How do I convert string math expression to an actual math …
Nov 4, 2016 · I'd recommend using a library such as Math.js as this will parse the math expression using a regular expression. This will avoid opening the application up to Cross-site scripting (XSS) attacks. minor thing here: Under the covers math.js uses Function().
Top 3 Effective Methods to Evaluate Mathematical Expressions
Nov 23, 2024 · Here is how you can use it to evaluate a string expression: const math = require('mathjs'); let expression = 'sin(45 deg) ^ 2'; let result = math.evaluate(expression); …
- Some results have been removed