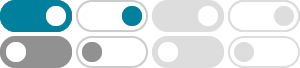
Get all unique values in a JavaScript array (remove duplicates)
The Array.from is useful to convert the Set back to an Array so that you have easy access to all of the awesome methods (features) that arrays have. There are also other ways of doing the same thing. But you may not need Array.from at all, as Sets have plenty of useful features like forEach.
How can I create a two dimensional array in JavaScript?
To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns. function Create2DArray(rows,columns) { var x = new Array(rows); for (var i = 0; i < rows; i++) { x[i] = new Array(columns); } return x; }
javascript - How to create an array containing 1...N - Stack Overflow
You can do so: var N = 10; Array.apply(null, {length: N}).map(Number.call, Number) result: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
How do I empty an array in JavaScript? - Stack Overflow
Aug 5, 2009 · Ways to clear an existing array A:. Method 1 (this was my original answer to the question) A = []; This code will set the variable A to a new empty array.
Javascript how to create an array of arrays - Stack Overflow
May 22, 2013 · Could somebody please help me to create an array of array.I have a matrix calculator and I want to create an array of arrays ('smaller').How can I do it?The functiions create2Darray and calculateDet are working ok,so now problem with them.Would be really grateful for the help.I need an array of these arrays (main matrix's minors) to calculate ...
What is the way of declaring an array in JavaScript?
The 2nd example makes an array of size 3 (with all elements undefined). The 3rd and 4th examples are the same, they both make arrays with those elements. Be careful when using new Array(). var x = new Array(10); // array of size 10, all elements undefined var y = new Array(10, 5); // array of size 2: [10, 5] The preferred way is using the ...
Best way to store a key=>value array in JavaScript?
In a language like php this would be considered a multidimensional array with key value pairs, or an array within an array. I'm assuming because you asked about how to loop through a key value array you would want to know how to get an object (key=>value array) like the person object above to have, let's say, more than one person.
javascript - How to convert Set to Array? - Stack Overflow
Here is an easy way to get only unique raw values from array. If you convert the array to Set and after this, do the conversion from Set to array. This conversion works only for raw values, for objects in the array it is not valid. Try it by yourself. let myObj1 = …
Is there an arraylist in Javascript? - Stack Overflow
Nov 17, 2009 · The last element of the array is removed from the array and returned..push ( [ item1 [ , item2 [ , … ] ] ] ) The arguments are appended to the end of the array, in the order in which they appear. The new length of the array is returned as the result of the call.".reverse ( ) The elements of the array are rearranged so as to reverse their order.
How to initialize an array's length in JavaScript?
Jan 31, 2011 · Defining JavaScript Arrays and Array Entries 1. JavaScript Array Entries. JavaScript pretty much has 2 types of array entries, namely mappable and unmappable. Both entry types are iterable, meaning they both work with the for-of loop. 2. JavaScript Arrays. A JavaScript array can contain both mappable and unmappable array entries.