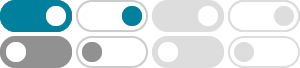
Python Program to Find LCM
The formula to calculate LCM is lcm(a, b) = abs(a*b) // gcd(a, b), where gcd() is the greatest common divisor of a and b. For example, with inputs 12 and 15, the output should be 60.
Python Program to Find LCM of Two Numbers - GeeksforGeeks
Jul 2, 2024 · In this example, the function LCM(a, b) calculates the Least Common Multiple (LCM) of two numbers a and b by iterating through multiples of the greater number within a range up to their product, and returns the first multiple that is divisible by the smaller number.
Python LCM: Understanding and Implementing the Least Common Multiple
Jan 20, 2025 · In Python, calculating the Least Common Multiple (LCM) can be achieved through different methods. The math library provides an efficient way to calculate the LCM using the relationship between the LCM and the GCD.
Calculate the LCM of a list of given numbers in Python
May 15, 2016 · I have written a code to find out the LCM (Lowest Common Multiple) of a list of numbers but there appears to be an error in my code. The code is given below: def final_lcm(thelist): previous_th...
Python Programs to Find HCF and LCM of two numbers - PYnative
Mar 31, 2025 · In Python, you can use the math module to find the Highest Common Factor (HCF) and the Least Common Multiple (LCM) of two numbers. The math module provides built-in functions math.gcd() for HCF (or GCD).
Find GCD and LCM in Python (math.gcd(), lcm()) - nkmk note
Aug 12, 2023 · This article explains how to find the greatest common divisor (GCD) and least common multiple (LCM) in Python.
Finding the Least Common Multiple (LCM) Using Python
Jan 30, 2025 · In this post, I will provide a Python code to find the LCM of a given set of numbers. The code uses the following formula to iteratively compute the LCM: where GCD stands for Greatest Common Divisor. The program uses Python’s math.gcd () function to compute the GCD of two numbers.
Python Program to Find LCM - Naukri Code 360
Aug 5, 2024 · To find the Least Common Multiple (LCM) of three numbers in Python, you can calculate the LCM of the first two numbers, and then compute the LCM of the result and the third number using the lcm() function.
Python Program To Find LCM Of Two Numbers - Unstop
To find the LCM of two numbers in Python, we can use the LCM formula inside a for loop, a while loop, or a recursive function. Alternatively, we can use the math module's built-in functions gcd () and lcm ().
ari-1/lcm.py — Python — NumWorks
Oct 30, 2024 · Finds the LCM of any number of integers. def gcd ( a , b ): while b : a , b = b , a % b return a def lcm ( a , b ): return a * b // gcd ( a , b ) def calculate_lcm ( numbers ): from functools import reduce return reduce ( lcm , numbers ) input_numbers = input ( " Enter numbers separated by commas: " ) numbers = list ( map ( int , input_numbers ...