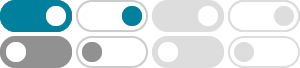
javascript - What is the difference between "let" and "var"? - Stack ...
Apr 18, 2009 · In other words,let implicitly hijacks any block's scope for its variable declaration. let variables cannot be accessed in the window object because they cannot be globally accessed. function a(){ { // this is the Max Scope for let variable let x = 12; } console.log(x); } a(); // Uncaught ReferenceError: x is not defined
JavaScript OR (||) variable assignment explanation
In summary, this technique is taking advantage of a feature of how the language is compiled. That is, JavaScript "short-circuits" the evaluation of Boolean operators and will return the value associated with either the first non-false variable value or whatever the last variable contains.
javascript - Declaring vs Initializing a variable? - Stack Overflow
Jul 30, 2015 · A variable declaration (e.g., var foo) causes that variable to be created as soon as the "lexical environment" is instantiated. For example, if that variable were defined within a function body, that function is the "lexical environment", and so variable creation coincides with the instantiation of the function itself.
Defining JavaScript variables inside if-statements
Because JavaScript's variables have function-level scope, your first example is effectively redeclaring your variable, which may explain why it is getting highlighted. On old versions of Firefox, its strict JavaScript mode used to warn about this redeclaration, however one of its developers complained that it cramped his style so the warning ...
What's the difference between variable definition and declaration …
Dec 29, 2013 · Is this a variable definition or declaration? And why? var x;..and is the memory reserved for x after this statement? EDIT: In C extern int x; is a declaration, int x = 5; is a definition. What's the analog in JS? Wikipedia says a declaration allocates memory and the definition assigns a value to this allocated memory.
Declaring a boolean in JavaScript using just var - Stack Overflow
Mar 26, 2013 · Furthermore, there are many possibilites: if you're not interested in exact types use the '==' operator (or ![variable] / !![variable]) for comparison (that is what Douglas Crockford calls 'truthy' or 'falsy' I think). In that case assigning true or 1 or '1' to the unitialized variable always returns true when asked.
javascript - Why does my `name` variable show it's deprecated?
Dec 20, 2020 · In a browser, the global name variable has special meaning. This has caused people a lot of confusion over the years as they tried to create their own global variable named name and then found it coerced into a string. The checker you are using doesn't appear able to special case an assignment to name if it follows a declaration of let name.
Best Way for Conditional Variable Assignment - Stack Overflow
Nov 11, 2019 · If the condition evaluates to false the condition on the right will be assigned to the variable. You can also nest many conditions into one statement. var a = (true)? "true" : "false"; Nesting example of method 1: Change variable A value to 0, 1, 2 and a negative value to see how the statement would produce the result. var a = 1; var b = a > 0?
In Javascript, can I use a variable before it is declared?
Mar 20, 2014 · Yes. In JavaScript, variables are hoisted. A variable statement declares variables that are created as defined in 10.5. Variables are initialised to undefined when created. A variable with an Initialiser is assigned the value of its AssignmentExpression when the VariableStatement is executed, not when the variable is created. ES5 §12.2
Declaring javascript variables as specific types - Stack Overflow
Quite simply, JavaScript variables do not have types. The values have types. The language permits us to write code like this: var foo = 42; foo = 'the answer'; foo = function {}; So it would be pointless to specify the type in a variable declaration, because the type is dictated by the variable's value. This fairly common in "dynamic" languages.