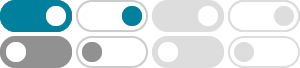
How do shift operators work in Java? - Stack Overflow
Right and Left shift work on same way here is How Right Shift works; The Right Shift: The right shift operator, >>, shifts all of the bits in a value to the right a specified number of times. Its general form: value >> num Here, num specifies the number of …
java - Difference between >>> and - Stack Overflow
May 11, 2010 · >> Signed right shift >>> Unsigned right shift The bit pattern is given by the left-hand operand, and the number of positions to shift by the right-hand operand. The unsigned right shift operator >>> shifts a zero into the leftmost position, while the leftmost position after >> depends on sign extension.
What is the purpose of the unsigned right shift operator ">>>" in …
Mar 18, 2015 · With the unsigned-right-shift operator, however, the most significant bit ends up being interpreted just like any other. The unsigned right-shift operator may also be useful when a computation would, arithmetically, yield a positive number between 0 and 4,294,967,295 and one wishes to divide that number by a power of two.
How does bitshifting work in Java? - Stack Overflow
When you shift right 2 bits you drop the 2 least significant bits. So: x = 00101011 x >> 2 // now (notice the 2 new 0's on the left of the byte) x = 00001010 This is essentially the same thing as dividing an int by 2, 2 times. In Java. byte b = (byte) 16; b = b >> 2; // prints 4 System.out.println(b);
What are bitwise shift (bit-shift) operators and how do they work?
Unsigned Right Shift(>>>): This operator also shifts bits to the right. The difference between signed and unsigned is the latter fills the leading bits with 1 if the number is negative and the former fills zero in either case. Now the question arises why we need unsigned right operation if we get the desired output by signed right shift operator.
unsigned right Shift '>>>' Operator in Java - Stack Overflow
Jan 24, 2013 · If the promoted type of the left-hand operand is int, only the five lowest-order bits of the right-hand operand are used as the shift distance. It is as if the right-hand operand were subjected to a bitwise logical AND operator & (§15.22.1) with the mask value 0x1f (0b11111).
What does the ">>" symbol mean in Java? - Stack Overflow
May 2, 2015 · The bit pattern is given by the left-hand operand, and the number of positions to shift by the right-hand operand. The unsigned right shift operator >>> shifts a zero into the leftmost position, while the leftmost position after >> depends on sign extension. (12 >> 1) - 1) >> shifts binary 12(1100) 1 times to the right. 12 >> 1 == 6(110)
Why do we need to use shift operators in java? - Stack Overflow
The shift operator is used when you're performing logical bits operations, as opposed to mathematical operations. It can be used for speed, being significantly faster than division/multiplication when dealing with operands that are powers of two, but clarity of code is usually preferred over raw speed.
java - Bitwise shift operators. Signed and unsigned - Stack Overflow
May 17, 2015 · The << operator doesn't actually preserve the sign, as you suggest; it simply happens to in your example. Try doing a left shift on 2,147,483,647; it doesn't stay positive. The reason that they don't bother trying to make a 'signed' left shift is because, if the number shifts from positive to negative (or viceversa), then you went outside the ...
Java shift operator - Stack Overflow
Feb 9, 2012 · I was wrong, only right shift operator's ignoring the sign bit. 10100000 << 1 == 01000000 For unsigned right shifting there's an operator >>> which shifts sign bit too. 11000000>>1 == 10100000 and 11000000>>>1 == 01100000