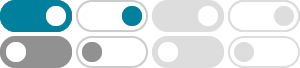
Creating an array that stores strings and integers in java
Dec 11, 2014 · I would like to create an array that stores the names (String) and values (integer) of company stocks, but I don't know how to go about it. Any tries by yourself? Create a class to hold these attributes and then create an array to hold instances of this class.
How do I declare and initialize an array in Java? - Stack Overflow
Jul 29, 2009 · Declare and initialize for Java 8 and later. Create a simple integer array: int [] a1 = IntStream.range(1, 20).toArray(); System.out.println(Arrays.toString(a1)); // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19] Create a random array for integers between [-50, 50] and for doubles [0, 1E17]:
Java Arrays - W3Schools
Java Arrays. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type with square brackets:
java - Converting String Array to an Integer Array - Stack Overflow
Converting String array into stream and mapping to int is the best option available in java 8. String[] stringArray = new String[] { "0", "1", "2" }; int[] intArray = Stream.of(stringArray).mapToInt(Integer::parseInt).toArray(); System.out.println(Arrays.toString(intArray));
String Arrays in Java - GeeksforGeeks
Nov 25, 2024 · When we create an array of type String in Java, it is called a String Array in Java. In this article, we will learn the concepts of String Arrays in Java including declaration, initialization, iteration, searching, sorting, and converting a String Array to a single string.
Arrays in Java - GeeksforGeeks
Mar 28, 2025 · To declare an array in Java, use the following syntax: type [] arrayName; type: The data type of the array elements (e.g., int, String). arrayName: The name of the array. Note: The array is not yet initialized. 2. Create an Array. To create an array, you need to allocate memory for it using the new keyword:
Java Program to Convert String to Integer Array - GeeksforGeeks
Nov 19, 2024 · There are 2 methods to convert a String to an Integer Array, which are as follows: 1. Using replaceAll () and split () methods (for Strings with Brackets or Other Delimiters like Commas) The string.replaceAll () method takes two arguments, a …
Java How To Convert a String to an Array - W3Schools
There are many ways to convert a string to an array. The simplest way is to use the toCharArray() method: Convert a string to a char array: You can also loop through the array to print all array elements:
How to create a String or int Array in Java? Example Tutorial
There are three main ways to create a String array in Java, e.g. here is a String array with values : String [ ] platforms = { "Nintendo" , "Playstation" , "Xbox" } ; and here is a String array without values :
Initializing Arrays in Java - Baeldung
Dec 16, 2024 · int[] array = new int[5]; Arrays.fill(array, 0, 3, -50); Here, the fill() method accepts the initialized array, the index to start the filling, the index to end the filling (exclusive), and the value itself respectively as arguments.