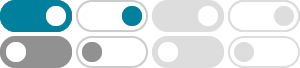
python - How do I call a function from another .py file ... - Stack ...
Define import statement in Example3.py - file for import all function. from com.my.func.DifferentFunction import * or define each function name which you want to import. from com.my.func.DifferentFunction import add, sub, mul Then in Example3.py you can call function for execute:
In Python, what happens when you import inside of a function?
Aug 24, 2016 · The one potentially substantial advantage of having the import within a function is when that function may well not be needed at all in a given run of the program, e.g., that function deals with errors, anomalies, and rare situations in general; if that's the case, any run that does not need the functionality will not even perform the import ...
module - Import functions in Python - Stack Overflow
Apr 27, 2019 · import functions from functions import a, b, c functions.x = 1 functions.y = 2 functions.z = 3 print(a()) print(b()) print(c()) Because Python is lexically scoped, each function keeps a reference to the global scope in which it was defined. Name lookups use that scope to resolve free variables.
python - Use 'import module' or 'from module import ... - Stack …
Oct 28, 2014 · Since I am also a beginner, I will be trying to explain this in a simple way: In Python, we have three types of import statements which are: 1. Generic imports: import math this type of import is my personal favorite, the only downside to this import technique is that if you need use any module's function you must use the following syntax: math ...
python - How to use the __import__ function to import a name …
Mar 21, 2012 · Hmm, you should be able to import foo (if you know it's value already and don't need to import it dynamically as a string value) with the normal import statement. Once the module is imported you can import anything within its directory as a string using getattr. import foo bar = getattr(foo, 'bar') object=bar.object –
Importing from a relative path in Python - Stack Overflow
Python has a concept of packages, which is basically a folder containing one or more modules, and zero-or-more packages. When we launch python, there are two ways of doing it: Asking python to execute a specific module (python3 path/to/file.py). Asking python to execute a package. The issue is that import.py makes reference to importing .math
python - Importing files from different folder - Stack Overflow
By default, you can't. When importing a file, Python only searches the directory that the entry-point script is running from and sys.path which includes locations such as the package installation directory (it's actually a little more complex than this, but this covers most cases).
python - Dynamically import a method in a file, from a string
Jan 9, 2012 · From Python 2.7 you can use the importlib.import_module() function. You can import a module and access an object defined within it with the following code: from importlib import import_module p, m = name.rsplit('.', 1) mod = import_module(p) met = …
python - Importing a function from a class in another file? - Stack ...
Note - the parts in capital letters are where you type the file name and function name. Now you just use your function however it was meant to be. Example: FUNCTION SCRIPT - saved at C:\Python27 as function_choose.py. def choose(a): from random import randint b = randint(0, len(a) - 1) c = a[b] return(c) SCRIPT USING FUNCTION - saved wherever
python - Importing modules from parent folder - Stack Overflow
Apr 3, 2009 · My code adds a file path to sys.path, the Python path list because this allows Python to import modules from that folder. After importing a module in the code, it's a good idea to run sys.path.pop(0) on a new line when that added folder has a module with the same name as another module that is imported later in the program. You need to remove ...