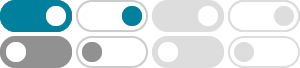
python - How do I make a time delay? - Stack Overflow
Notice in recent Python versions (Python 3.4 or higher) you can use asyncio.sleep. It's related to asynchronous programming and asyncio. It's related to asynchronous programming and asyncio. Check out next example:
How do I get my program to sleep for 50 milliseconds?
Nov 1, 2024 · Sleep asks the program to wait, and then to do the rest of the code. There are two ways to use sleep: import time # Import whole time module print("0.00 seconds") time.sleep(0.05) # 50 milliseconds... make sure you put time. if you import time! print("0.05 seconds") The second way doesn't import the whole module, but it just sleep.
How accurate is python's time.sleep ()? - Stack Overflow
If secs is zero, Sleep(0) is used. Unix implementation: Use clock_nanosleep() if available (resolution: 1 nanosecond); Or use nanosleep() if available (resolution: 1 nanosecond); Or use select() (resolution: 1 microsecond). So just calling time.sleep will be fine on most platforms starting from python 3.11, which is a great news ! It would be ...
python - asyncio.sleep () vs time.sleep () - Stack Overflow
However, the main difference is that time.sleep(5) is blocking, and asyncio.sleep(5) is non-blocking. When time.sleep(5) is called, it will block the entire execution of the script and it will be put on hold, just frozen, doing nothing.
python - time.sleep -- sleeps thread or process? - Stack Overflow
Sep 18, 2008 · akki, More specifically, time.sleep() blocks the thread that called time.sleep(), but it releases the Python GIL to run other threads (so it doesn't block the process). Nick's example didn't really show the blocking of the thread, it more showed that the GIL is released (thus showing that the process IS NOT blocked).
How to sleep Selenium WebDriver in Python for milliseconds
import time time.sleep(1) #sleep for 1 sec time.sleep(0.25) #sleep for 250 milliseconds However while using Selenium and WebDriver for Automation using time.sleep(secs) without any specific condition to achieve defeats the purpose of Automation and should be avoided at any cost. As per the documentation:
Display a countdown for the python sleep function
time.sleep() may return earlier if the sleep is interrupted by a signal or later (depends on the scheduling of other processes/threads by OS/the interpreter). To improve accuracy over multiple iterations, to avoid drift for large number of iterations, the …
sleep - Correct way to pause a Python program - Stack Overflow
Nov 8, 2020 · For a long block of text, it is best to use input() (or raw_input() on Python 2.x) to prompt the user, rather than a time delay. Fast readers won't want to wait for a delay, slow readers might want more time on the delay, someone might be interrupted while reading it and want a lot more time, etc.
In Python, how can I put a thread to sleep until a specific time?
Aug 25, 2020 · If you just want to sleep until whatever happens to be the next 2AM, (might be today or tomorrow), you need an if-statement to check if the time has already passed today. And if it has, set the wake up for the next day instead. import time sleep_until = "02:00AM" # Sets the time to sleep until.
What's the most efficient way to sleep in Python?
Jul 5, 2016 · $ ipython import time,select %timeit time.sleep(0) 1000000 loops, best of 3: 655 ns per loop %timeit select.select([],[],[],0) 1000000 loops, best of 3: 902 ns per loop But if you don't have access to ipython, and would prefer native timeit from command line: