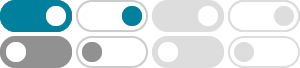
C++ Classes and Objects - W3Schools
A class is a user-defined data type that we can use in our program, and it works as an object constructor, or a "blueprint" for creating objects. Create a Class To create a class, use the class keyword:
C++ Classes and Objects - GeeksforGeeks
Mar 20, 2025 · In C++, classes and objects are the basic building block that leads to Object-Oriented programming in C++. We will learn about C++ classes, objects, look at how they work and how to implement them in our C++ program. What is a Class?
15.2 — Classes and header files – Learn C++ - LearnCpp.com
Jan 4, 2025 · Putting class definitions in a header file. If you define a class inside a source (.cpp) file, that class is only usable within that particular source file. In larger programs, it’s common that we’ll want to use the classes we write in multiple source files.
C++ Classes and Objects (With Examples) - Programiz
In this tutorial, we will learn about objects and classes in C++ with the help of examples. Objects and classes are used to wrap the related functions and data in one place in C++.
14.2 — Introduction to classes – Learn C++ - LearnCpp.com
Jun 26, 2024 · Most of the C++ standard library is classes. You have already been using class objects, perhaps without knowing it. Both std::string and std::string_view are defined as classes. In fact, most of the non-aliased types in the standard library are defined as classes!
Classes (I) - C++ Users
Classes are defined using either keyword class or keyword struct, with the following syntax: class class_name { access_specifier_1: member1; access_specifier_2: member2; ... } object_names;
How to Use Classes in C++: A Quick Guide - cppscripts.com
Master the art of how to use classes in C++. This concise guide unveils the essentials of object-oriented programming for your coding journey. In C++, classes are user-defined data types that encapsulate data and functions, enabling the creation of objects; the following example demonstrates a simple class definition and object instantiation:
C++ Classes and Objects - Online Tutorials Library
A class is used to specify the form of an object and it combines data representation and methods for manipulating that data into one neat package. The data and functions within a class are called members of the class. C++ Class Definitions. When you define a …
Learn C++ Classes: Definition, Examples, and How to Use Them
Mar 21, 2025 · To declare a class in C++, you use the class keyword followed by the name of the class. For example: // class members. To define a class in C++, you need to specify its members, which include data members and member functions. For example: int x; // data member. void myFunction(); // member function.
Mastering Classes in CPP: A Quick Guide
How to Use Classes in C++. Once created, using a class involves instantiating objects from it. This is a straightforward process: Student student1; student1.setDetails("Alice", 20); student1.displayInfo(); In this example, an object `student1` is created from the `Student` class, and methods are called to set and display the student’s ...