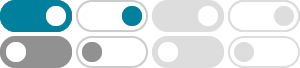
java - How to store arrays in single array - Stack Overflow
To access a single element of the selected array you need to do something like: use the following syntax. Create array-of-arrays: int[] array1 = {1,2,3,4,5,100,200,400}; . int[] array2 = {2,6,5,7,2,5,10}; int[] array3 = {11,65,4,3,2,9,7}; int[] array4 = {111,33,22,55,77}; int[][] allArrays = { . array1, array2, array3, array4. };
java - How can I store multiple variable values into a single array …
Below is a demonstration of how I want the data to be stored. Kindly provide me with the simplest solution as possible. Thanks. Use a class and then a arraylist of that class. You could use an ArrayList<? extends Object> and then cast from Object to the correct type for each item index.
Java Program to Merge Two Arrays - GeeksforGeeks
Nov 14, 2024 · Given two arrays, the task is to merge or concatenate them and store the result into another array. Example: Explanation: The simplest and most efficient way to merge two arrays is by using the in-built System.arraycopy() function. First, we initialize two arrays, a and b, and store values in them.
Can you store multiple integers at one array index?
Nov 30, 2012 · Yes, it is possible to add multiple int to an array, but you would need to have an array where each item is an Object rather than an int. For example... int number1 = -1; int number2 = -1; int number3 = -1; public void addInt(int number){ if (number1 == -1){ number1 = number; else if (number2 == -1){ number2 = number; else if (number3 == -1){
Arrays — store multiple items using the same variable.
Jun 28, 2021 · Here, two ‘for loops’ are used: one for storing the array values and the other one for displaying the values. Loops can be used to work with arrays efficiently. These arrays are 1-dimensional.
Concatenate the Array of elements into a single element
Dec 6, 2023 · Given an array arr[] of length N, the task for every array element is to print the sum of its Bitwise XOR with all other array elements. Examples: Input: arr[] = {1, 2, 3}Output: 5 4 3Explanation:For arr[0]: arr[0] ^ arr[0] + arr[0] ^ arr[1] + arr[0] ^ …
C Program To Merge Two Arrays - GeeksforGeeks
Aug 30, 2024 · Merging two arrays means combining/concatenating the elements of both arrays into a single array. Example. Input: arr1 = [1, 3, 5], arr2 = [2, 4, 6] Output: res = [1, 3, 5, 2, 4, 6] Explanation: The elements from both arrays are merged into a single array. Input: arr1 = [10, 40, 30], arr2 = [15, 25, 5] Output: res = [10, 40, 30, 15, 25, 5]
Array in Java: store multiple values in a single variable
An array in Java is used to store multiple values in a single variable, instead of declaring separate variables for each value. In other words, an array is a collection of elements stored in what can be described as a list. Each element in an array consists of the same data type and can be retrieved and used with its index.
Storing multiple objects in a single array element in Java
Sep 17, 2020 · You can store anything in an Object[] object array. Something like this: Object[] objArr = new Object[10]; objArr[0] = "String"; objArr[1] = Arrays.asList(1, 2, 4); objArr[2] = new ArrayList<>(); objArr[3] = 1;
How to store 2 elements in one array - C++ Forum - C++ Users
Jan 9, 2018 · But in case it isn't clear, you can declare an array like this: int arrayName[2]; // array that can hold 2 ints