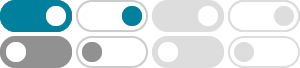
Best way to check that a variable is only an integer in JavaScript
Nov 19, 2012 · Use isNaN (is not a number - but beware that the logic is negated) and combine with parseInt: function is_int(x) { return (!isNaN(x) && parseInt(x) == x) } As suggested here, the following will also do: function isInt(n) { return n % 1 === 0; }
JavaScript Number isInteger() Method - W3Schools
The Number.isInteger() method returns true if a value is an integer of the datatype Number. Otherwise it returns false.
How to use Scanner to accept only valid int as input
Use Scanner.hasNextInt(): Returns true if the next token in this scanner's input can be interpreted as an int value in the default radix using the nextInt() method. The scanner does not advance past any input. Here's a snippet to illustrate: while (!sc.hasNextInt()) sc.next(); num2 = sc.nextInt();
javascript - How can I tell is integer one or two digit number?
Mar 16, 2015 · The easiest check is to divide the number by 10, and add a leading zero if it's output is between 0 and 1. var a = numberYouWant; if(a/10 < 1 && a/10 > 0){ a = "0" + String(a); } Share
Number.isInteger() - JavaScript | MDN - MDN Web Docs
Mar 5, 2025 · The Number.isInteger() static method determines whether the passed value is an integer. Try it function fits(x, y) { if (Number.isInteger(y / x)) { return "Fits!"; } return "Does NOT fit!"; } console.log(fits(5, 10)); // Expected output: "Fits!"
Number Validation in JavaScript - GeeksforGeeks
Jan 3, 2025 · Validating a number string in JavaScript involves ensuring that a given string represents a valid number. This typically includes checking for digits, optional signs (+/-), decimal points, and possibly exponent notation (e.g., "1.23e4").
How to check for a number in JavaScript
Sep 23, 2019 · Checking for an integer specifically involves using the parseInt() function, followed by comparing the parsed value to itself using == (which will type coerce non-number values, such as strings to numbers) or === (which will only return true if both values are numbers).
3 Ways to Check if a Value is a Number in JavaScript
May 18, 2024 · In this tutorial, we'll explore three different ways to check if a value is a number in JavaScript, using the isInteger(), typeof(), and isNaN() methods. The isInteger() method in JavaScript accepts a single parameter, the value being tested. The method returns true if the value is numeric, and false if it isn't:
JavaScript Number isInteger() Method: Checking Integer
Feb 5, 2025 · A comprehensive guide to the JavaScript Number.isInteger() method, including syntax, examples, and practical use cases for checking if a value is an integer.
JavaScript: Check for integer values using the isInteger() method
Apr 1, 2022 · JavaScript Number object comes with the isInteger() method that you can use to check if the passed argument is a whole number (integer). The method accepts one parameter: the value to be tested if it’s an integer.
- Some results have been removed