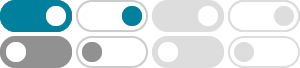
How do I save and restore multiple variables in python?
Jul 16, 2020 · If you need to save multiple objects, you can simply put them in a single list, or tuple, for instance: # obj0, obj1, obj2 are created here... pickle.dump([obj0, obj1, obj2], f) obj0, obj1, obj2 = pickle.load(f)
How to Save a Variable in a File in Python - Python Pool
Apr 23, 2021 · Ways to save a variable in a file in python. 1. Using string concatenation to save a variable in a file in Python; 2. Using String formatting to save a variable in a file in Python; 3. By importing pickle library to save a variable in a file; 4. Using …
How to use Pickle to save and load Variables in Python?
Feb 25, 2021 · This article discusses how variables can be saved and loaded in python using pickle. Functions used: In python, dumps() method is used to save variables to a pickle file. Syntax: pickle.dumps(obj, protocol=None, *, fix_imports=True, buffer_callback=None) In python, loads() is used to load saved data from a pickled file; Syntax:
How to Save Variables to a File in Python? - Python Guides
Feb 14, 2025 · I explained how to save a single variable to a Python file, save multiple variables to a Python file, use string formatting, write variables on separate lines, and save variables with file name from user input.
How to Write a Variable to a File in Python? - Python Guides
Sep 19, 2024 · In this tutorial, I explained how to write variables to a file in Python using different methods, such as using write(), writelines(), print(), etc. I have also provided some examples of adding different variable types to a file in Python.
saving and reloading variables in Python, preserving names
Feb 19, 2021 · You can save the variables, using e.g. numpy.save, but it doesn't preserve the variable names. So unless you know the order you saved them in you can't recreate them. I want them as separate variables, not just as part of an array …
Save variable value in python - Stack Overflow
May 2, 2013 · The best you can do is to use a global variable: global counter. counter += 1. print counter. An alternative which bypasses the need for the global statement would be something like: print next(counter) . or even: print next(_counter)
Write Multiple Variables to a File using Python - GeeksforGeeks
Apr 12, 2025 · Storing multiple variables in a file is a common task in programming, especially when dealing with data persistence or configuration settings. In this article, we will explore three different approaches to efficiently writing multiple variables in a file using Python.
Saving Text, JSON, and CSV to a File in Python | GeeksforGeeks
Dec 29, 2020 · To pass a variable-length key-value pair as an argument to a function, Python provides a feature called **kwargs.kwargs stands for Keyword arguments. It proves to be an efficient solution when one wants to deal with named arguments (arguments passed with a specific name (key) along with their value)
Best Ways to Save Data in Python - AskPython
Feb 26, 2021 · 1. Using Pickle to store Python Objects. If we want to keep things simple, we can use the pickle module, which is a part of the standard library to save data in Python. We can “pickle” Python objects to a pickle file, which we can use to save/load data. So if you have a custom object which you might need to store / retrieve, you can use ...