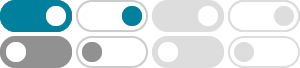
How to reverse user input in Python - Stack Overflow
Mar 17, 2015 · I am working on a script that takes the user input and reverses the whole input. for example if the user inputs "London" it will be printed as "nodnol" . I am currently being able to reverse the order of a certain number of letters but not being able to reverse the entire string .
How to Reverse a String in Python - W3Schools
There is no built-in function to reverse a String in Python. The fastest (and easiest?) way is to use a slice that steps backwards, -1. Reverse the string "Hello World": We have a string, "Hello World", which we want to reverse: Create a slice that starts at …
How do I reverse a string in Python? - Stack Overflow
May 31, 2009 · There is no built-in reverse function in Python's str object. Here is a couple of things about Python's strings you should know: In Python, strings are immutable. Changing a string does not modify the string. It creates a new one. Strings are sliceable.
"Reverse" input in Python - Stack Overflow
Oct 9, 2012 · Simply split the string into a list (here I use ' ' as the split character), reverse it, and put it back together again: s = raw_input('Please type in your full name') ' '.join(reversed(s.split(' ')))
How to reverse a String in Python - GeeksforGeeks
Nov 21, 2024 · Python provides a built-in function called reversed (), which can be used to reverse the characters in a string. This approach is very useful if we prefer to use built-in methods. Explanation: reversed (s) returns an iterator of the characters in s in reverse order. ”.join (reversed (s)) then joins these characters into a new string.
Reverse Strings in Python: reversed(), Slicing, and More
In this step-by-step tutorial, you'll learn how to reverse strings in Python by using available tools such as reversed() and slicing operations. You'll also learn about a few useful ways to build reversed strings by hand.
Python reversed() Method - GeeksforGeeks
Mar 8, 2025 · reversed() function in Python lets us go through a sequence like a list, tuple or string in reverse order without making a new copy. Instead of storing the reversed sequence, it gives us an iterator that yields elements one by one, saving memory.
Python String Reversal
String reversal is a common programming task that can be approached in multiple ways in Python. This comprehensive guide will explore various methods to reverse a string, discussing their pros, cons, and performance characteristics.
Python Program For Reverse Of A String (6 Methods With Code)
To reverse a string using slicing in Python, you can utilize the slice notation with a step value of -1. Here’s an example. Python Program For Reverse Of A String def reverse_string(input_string): return input_string[::-1] string = "Hello, World!" reversed_string = reverse_string(string) print(reversed_string) Output
Python Reverse String - 5 Ways and the Best One - DigitalOcean
Aug 3, 2022 · Python String doesn’t have a built-in reverse () function. However, there are various ways to reverse a string in Python. 1. How to Reverse a String in Python? Some of the common ways to reverse a string are: Using Slicing to create a reverse copy of the string. return s[::-1] print('Reverse String using slicing =', reverse_slicing(input_str))