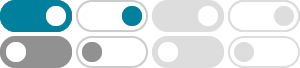
How to Remove an Element from Array in C++? - GeeksforGeeks
Oct 11, 2024 · To remove a value from an array in C++, we first search the position of the element to be removed, then move elements that are on the right side of this element to one position towards the front (i.e. towards the left). Then decrement the array size variable.
Remove an array element and shift the remaining ones
Dec 22, 2015 · int array[] = { 1,2,3,4,5 }; auto itr = std::remove(std::begin(array), std::end(array), 3); int newArray[4]; std::copy(std::begin(array), itr, std::begin(newArray));
Deleting Elements in an Array – Array Operations - GeeksforGeeks
Nov 9, 2024 · In this post, we will look into deletion operation in an Array, i.e., how to delete an element from an Array, such as: Delete an Element from the Beginning of an Array; Delete an Element from a Given Position in an Array; Delete First Occurrence of Given Element from an Array; Remove All Occurrences of an Element in an Array
Delete element from C++ array - Stack Overflow
Jul 11, 2015 · vector<int> remove_frist_x_items(const vector<int>& arr, int x) { return vector<int>(arr.begin() + x, arr.end()); } // Delete the first element from the array auto new_array = remove_frist_x_items(the_old_one, 1);
c++ - delete [] an array of objects - Stack Overflow
Mar 21, 2010 · delete &array[i]; will be deleting things that weren't returned by a new operation. Not to mention that the subsequent delete[] array; will call the destructor for all the objects that just had destructors called in the loop.
How to Delete an Element from an Array in C++? - GeeksforGeeks
Mar 18, 2024 · Find the index of the element you want to delete from the array. Shift all the elements after that index to the left. Update the size of the array after deletion if you are keeping it. The following program illustrates how to delete an element from an array in C++.
How to Remove Element From Array in C++ - Delft Stack
Mar 12, 2025 · One of the most common ways to remove an element from an array in C++ is by shifting the elements. This method involves finding the element you want to remove, then shifting all subsequent elements one position to the left. Here’s how you can do it: arr[j] = arr[j + 1]; } .
C++ How to Delete an Array element - Studytonight
Oct 31, 2020 · This tutorial explains how to Delete an Array element in C++ with complete program and output.
How to Remove Element from Array in C++ | Markaicode
Nov 7, 2024 · Using Vector’s erase () Method. The simplest way to remove an element from an array in C++ is using the vector container: std::vector<int> numbers = {1, 2, 3, 4, 5}; // Remove element at index 2. numbers.erase(numbers.begin() + 2); // Print results. for(int num : numbers) { std::cout << num << " "; return 0; 2.
How to Delete an Element from Array in C++ - CodeSpeedy
Solution of how to delete elements in an array without shifting elements and with shifting elements in C++, with explanation and source codes.
- Some results have been removed