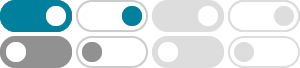
Remove whitespaces inside a string in javascript
May 29, 2012 · To remove any and/or all white space characters you should use: 's t r i n g'.replace(\s+\g, '') If the intention is to only remove specific types of whitespaces (ie. thin or hair spaces) they have to be listed explicitely like this:
JavaScript String trim() Method - W3Schools
The trim() method removes whitespace from both sides of a string. The trim() method does not change the original string.
javascript - Remove ALL white spaces from text - Stack Overflow
But, just for fun, you can also remove all whitespaces from a text by using String.prototype.split and String.prototype.join: Use string.replace(/\s/g,'')
How to remove spaces from a string using JavaScript?
May 11, 2011 · \s is the regex for "whitespace", and g is the "global" flag, meaning match ALL \s (whitespaces). A great explanation for + can be found here. As a side note, you could replace the content between the single quotes to anything you want, …
How to Trim Whitespaces/Characters from a String in JavaScript
May 22, 2023 · In this guide, learn how to trim the whitespaces at the start and end of a string in JavaScript with built-in methods and Regular Expressions. We'll use the `trim()`, `trimStart()`, `trimEnd()`, `substr()` and `replace()` methods.
JavaScript Program to Remove All Whitespaces From a Text
Oct 4, 2023 · In this article, we will see how to remove the white spaces from string using jQuery. To remove the white spaces, we will use trim() method. The trim() method is used to remove the white spaces from the beginning and end of a string. Syntax: jQuery.trim( str ) Parameter: This method accepts a single
Remove/Replace all Whitespace from a String in JavaScript
Mar 1, 2024 · Use the String.replace() method to remove all whitespace from a string, e.g. str.replace(/\s/g, ''). The replace() method will remove all whitespace characters from the string by replacing them with empty strings.
JavaScript trim () Method
In this tutorial, you'll learn how to use the JavaScript trim() method to remove whitespace characters from both ends of a string.
How to Remove Spaces From a String using JavaScript?
Nov 14, 2024 · How to Remove Spaces From a String using JavaScript? These are the following ways to remove space from the given string: 1. Using string.split () and array.join () Methods. JavaScript string.split () method is used to split a string into multiple sub-strings and return them in the form of an array.
javascript - How to trim () white spaces from a string? - Stack Overflow
Oct 13, 2008 · JavaScript doesn't seem to have a native trim() method. How can I trim white spaces at the start and end of a string with JavaScript?