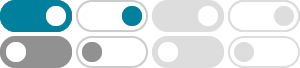
How to take character input in java - Stack Overflow
In C, we are able to take input as character with the keyword char from keyboard as scanf("%c", &ch); But In Java how to do this? I have tried this: import java.util.Scanner; public class...
java - Take a char input from the Scanner - Stack Overflow
Dec 19, 2012 · You can solve this problem, of "grabbing keyboard input one char at a time" very simply. Without having to use a Scanner all and also not clearing the input buffer as a side effect, by using this. char c = (char)System.in.read(); If all you need is the same functionality as the C language "getChar ()" function then this will work great.
How do I read input character-by-character in Java?
I am used to the c-style getchar(), but it seems like there is nothing comparable for java. I am building a lexical analyzer, and I need to read in the input character by character. I know I can use the scanner to scan in a token or line and parse through the token char-by-char, but that seems unwieldy for strings spanning multiple lines.
Java character input validation - Stack Overflow
Apr 21, 2015 · I want to validate a single character in a java application. I don't want the user to be able to enter a character outside the range [a - p] (ignoring uppercase or lowercase) or numbers. Scanner ...
How can I enter "char" using Scanner in java? - Stack Overflow
Apr 16, 2014 · I have a question how can I enter char using Scanner in java? Such as for integer number Scanner input=new Scanner(System.in); int a = input.nextInt(); but how can I do the same for char? thanks for helping.
Reading a single char in Java - Stack Overflow
Aug 29, 2010 · Here is a class 'getJ' with a static function 'chr ()'. This function reads one char. import java.io.InputStreamReader; import java.io.BufferedReader; import java.io.IOException; class getJ { static char chr()throws IOException{ BufferedReader bufferReader =new BufferedReader(new InputStreamReader(System.in)); return bufferReader.readLine().charAt(0); } } In order to read a char use this ...
Validating input using java.util.Scanner - Stack Overflow
Learn how to validate input using java.util.Scanner in Java with examples and best practices from Stack Overflow.
How do you prompt a user for an input in java - Stack Overflow
Nov 7, 2014 · Having imported java.util.Scanner, to get input from the user as a String, create a Scanner object that parameterizes System.in and assign userChoice the value of nextLine () invoked by the Scanner object: Scanner input = new Scanner(System.in); String userChoice = input.nextLine(); A few things about your code.
char - nextChar () in java - Stack Overflow
Why do you think getting a String input is inefficient? What do you think is slower or less efficient, Java's getting in a String and extracting a char or the user's typing in a char or chars?
Checking if a character is a special character in Java
My program asks the user to input the name of a file, and the program reads the text in the file and determines how many blanks spaces, digits, letters, and special characters are in the text. I have the code completed to determine the blanks, digits, and letters, however am unsure of how to check if a character is a special character.