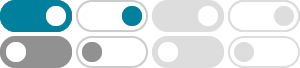
JavaScript Arrays - W3Schools
Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [item1, item2, ...]; It is a common practice to declare arrays with the const keyword. Learn more about const with arrays in the chapter: JS Array Const. Spaces and line breaks are not important. A declaration can span multiple lines:
What is the way of declaring an array in JavaScript?
There are a number of ways to create arrays. The traditional way of declaring and initializing an array looks like this: var a = new Array(5); // declare an array "a", of size 5 a = [0, 0, 0, 0, 0]; // initialize each of the array's elements to 0 Or... // declare and initialize an array in a single statement var a = new Array(0, 0, 0, 0, 0);
How to Declare an Array in JavaScript? - GeeksforGeeks
Oct 22, 2024 · There are varous ways to declare arrays in JavaScript, but the simplest and common is Array Litral Notations. The basic method to declare an array is using array litral notations. Use square bracket [] to declare an array and enclose comma-separated elements for initializing arrays.
How to Declare and Initialize an Array in JavaScript - W3docs
JavaScript allows declaring an Array in several ways. Let's consider two most common ways: the array constructor and the literal notation. The Array () constructor creates Array objects. You can declare an array with the "new" keyword to instantiate the array in memory. Here’s how you can declare new Array () constructor:
JavaScript Arrays - GeeksforGeeks
Feb 10, 2025 · Arrays in JavaScript are zero-indexed, meaning the first element is at index 0, the second at index 1, and so on. 1. Create Array using Literal. Creating an array using array literal involves using square brackets [] to define and initialize the array. 2. …
How to initialize an array in JavaScript - GeeksforGeeks
Oct 22, 2024 · Initializing an array in JavaScript involves creating a variable and assigning it an array literal. The array items are enclosed in square bracket with comma-separated elements. These elements can be of any data type and can be omitted for an empty array.
How to Declare an Array in JavaScript – Creating an Array in JS
Nov 14, 2022 · There are two standard ways to declare an array in JavaScript. These are either via the array constructor or the literal notation. In case you are in a rush, here is what an array looks like declared both ways: // Using array constructor let array = new array("John Doe", 24, true); // Using the literal notation let array = ["John Doe", 24, true];
JavaScript Arrays - How to Create an Array in JavaScript
May 16, 2022 · In this article I showed you three ways to create an array using the assignment operator, Array constructor, and Array.of() method. The most common way to create an array in JavaScript would be to assign that array to a variable like this:
How to Declare an Array in JavaScript - Expertbeacon
Aug 24, 2024 · Declaring Arrays in JavaScript. While array literal notation is the standard, newer syntaxes offer unique initialization approaches: Array Literal Notation. Literal notation with square brackets [] remains the simplest way to declare an array: let fruits = ["apple", "banana", "orange"]; Easy array visualization just by glancing at the syntax.
JavaScript Array
This tutorial introduces you to JavaScript array type and demonstrates the unique characteristics of JavaScript arrays via examples.
- Some results have been removed