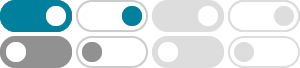
Binary Tree in C++ - GeeksforGeeks
Jul 3, 2024 · Implementation of Binary Tree in C++. A binary tree is a tree-based hierarchical data structure where each node can have at most two children. The topmost node is called the root, and nodes with no children are called leaves. To implement a binary tree in C++, we'll use a node-based approach.
Trees in C++ - Code of Code
In order to create a binary tree in C++, we must first create a class that will represent the nodes in the tree. Each node will have a value and two pointers, one for the left child and one for the right child. The following is an example of how to create a Node class in C++: Node *left, *right; Node(int data) { this->data = data; .
How to make a tree in C++? - Stack Overflow
Aug 10, 2011 · How do I make a tree data structure in C++ that uses iterators instead of pointers? I couldn't find anything in the STL that can do this. What I would like to do is to be able to create and manipulate trees like this: tree<int> myTree; tree<int>::iterator i = myTree.root(); *i = 42; tree<int>::iterator j = i.add_child(); *j = 777; j = j.parent();
Create a tree in level order - GeeksforGeeks
Sep 2, 2022 · Given an array of elements, the task is to insert these elements in level order and construct a tree. Input : arr[] = {10, 20, 30, 40, 50, 60} Output : 10 / \ 20 30 / \ / 40 50 60. The task is to construct a whole tree from a given array.
data structures - How to create a tree in C++? - Stack Overflow
Oct 7, 2016 · I want to create a tree in C++. I have the parent-child relationships and the number of nodes and I want to store this tree somehow. For example, if I have a graph, I can store it with adjacency lists, or using a vector of vectors, or a matrix of adjacency. But how about trees?
How to Build Binary Tree in C++ with example - CodeSpeedy
Mar 25, 2022 · Learn the basics of binary tree in C++. Create binary tree and learn how to traverse a binary tree in C++ with code snippet.
Trees in C++: A Beginner’s Guide to Understanding and …
Sep 26, 2024 · In this blog, we’ll delve into trees in C++, explain what makes them special, and how you can use them efficiently in your code.
Tree C/C++ Programs - GeeksforGeeks
May 22, 2024 · Various types such as binary tree, trie, etc. have different characteristics to make them suitable for different applications such as storing sorted data, dictionaries, routing tables, etc. In this article, we will discuss some top C/C++ practice problems on the tree data structure.
how to build a tree structure in C++ using std::map
May 20, 2012 · I am trying to write a tree sort of structure in C++. As in every tree there are branches and leaves. A branch can contain other branches as well as leaves. Now my implementation calls for each branch and leaf to have different functionalities. So for example. Take the tree structure. Root .
Tree Implementation in C++ - Tpoint Tech - Java
Mar 17, 2025 · The TreeNode class and the BinaryTree class are normally the two primary components that are defined in order to create a binary tree in C++. 1. TreeNode Class: A single node within a binary tree is represented by the TreeNode class.