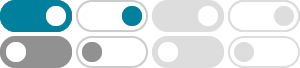
python - How to count the frequency of the elements in an …
Counting the frequency of elements is probably best done with a dictionary: b = {} for item in a: b[item] = b.get(item, 0) + 1 To remove the duplicates, use a set: a = list(set(a))
Counting the Frequencies in a List Using Dictionary in Python
Feb 10, 2025 · Counting the frequencies of items in a list using a dictionary in Python can be done in several ways. For beginners, manually using a basic dictionary is a good starting point. It uses a basic dictionary to store the counts, and the logic …
How to Count the Frequency of Elements in a Python List? - Python …
Mar 4, 2025 · Learn how to count the frequency of elements in a Python list using `Counter` from `collections`, `dict.get()`, and loops. This guide includes examples.
Python – List Frequency of Elements - GeeksforGeeks
Feb 10, 2025 · Counter (n) creates a frequency dictionary where each key is a unique element, and its value represents count of occurrences. This method is efficient and concise for counting elements in a list making it useful for frequency analysis.
Item frequency count in Python - Stack Overflow
May 21, 2009 · words = input().split(" ") for word in words: word_count = 0 for word2 in words: if word2.lower() == word.lower(): word_count += 1 print(f'{word} {word_count}')
Count the Frequency of Elements in a List
Aug 20, 2021 · We can count the frequency of elements in a list using a python dictionary. To perform this operation, we will create a dictionary that will contain unique elements from the input list as keys and their count as values.
What is the cleanest way to count frequencies in python
freq = Counter((int(round(x)) - 1) // 10 + 1 for x in data) will give correct bucket numbers... You could use numpy.histogram, which tabulates the frequencies at which elements in your data appear in a set of intervals (bins). It returns the counts in each bin and the rightmost edge of each bin: 70.03, 80.02, 90.01, 100. ])
How to Count Frequencies in a List in Python | SourceCodester
Mar 13, 2025 · Learn how to count frequencies in a list using Python. This step-by-step tutorial will guide you through the process to accurately count each element’s frequency.
3 Ways to Count the Item Frequencies in a Python List
Nov 2, 2022 · To get the frequency of all items at once, we can use one of the following two ways. The counter function in the collections module can be used for finding the frequencies of items in a list. It is a dictionary subclass and used for counting hashable objects.
5 Best Ways to List Frequency of Elements in Python
Mar 9, 2024 · By converting the list to a pandas.Series object, we can directly use the value_counts() method to obtain the frequency of each element. The result is a Series with the frequency of the elements sorted in descending order.