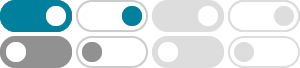
How to convert a string to an integer in JavaScript?
There are two main ways to convert a string to a number in JavaScript. One way is to parse it and the other way is to change its type to a Number. All of the tricks in the other answers (e.g., unary plus) involve implicitly coercing the type of the string to a number. You can also do the same thing explicitly with the Number function. Parsing const parsed = parseInt("97", 10); parseInt and ...
Javascript: Converting String to Number? - Stack Overflow
May 16, 2010 · Thanks, Fyodor. I'm trying to extract the number from a string using a regexp, and it returns a string, so I would want to convert it to a number so that it can be processed.
JavaScript string and number conversion - Stack Overflow
Below is a very irritating example of how JavaScript can get you into trouble: If you just try to use parseInt() to convert to number and then add another number to the result it will concatenate two strings.
Javascript to convert string to number? - Stack Overflow
For your case, just use: var str = '0.25'; var num = +str; There are some ways to convert string to number in javascript. The best way: var num = +str; It's simple enough and work with both int and float num will be NaN if the str cannot be parsed to a valid number You also can: var num = Number(str); //without new. work with both int and float or var num = parseInt(str,10); //for integer ...
What's the fastest way to convert String to Number in JavaScript?
Also, you might consider including string literal floats in the test for general comparison. My guess is that it may change the order in some cases because string to float conversion is generally slower than string to int conversion. The way the test is now, it's getting away with a string to int conversion when Number () is used.
What's the best way to convert a number to a string in JavaScript ...
The below are the methods to convert an Integer to String in JS. The methods are arranged in the decreasing order of performance in chrome. var num = 1 Method 1: num = `${num}` Method 2: num = num + '' Method 3: num = String(num) Method 4: num = num.toString()
javascript - How to convert a string to number - Stack Overflow
I can't figure it out how to convert this string 82144251 to a number. Code: var num = "82144251"; If I try the code below the .toFixed () function converts my number back to a string...
javascript - How to convert a string of numbers to an array of …
Mar 28, 2013 · backquote is string litteral so we can omit the parenthesis (because of the nature of split function) but it is equivalent to split(','). The string is now an array, we just have to map each value with a function returning the integer of the string so x=>+x (which is even shorter than the Number function (5 chars instead of 6)) is equivalent to :
javascript - How to convert a string to number in TypeScript?
String to number conversion: In Typescript we convert a string to a number in the following ways: parseInt(): This function takes 2 arguments, the first is a string to parse. The second is the radix (the base in mathematical numeral systems, e.g. 10 for decimal and 2 for binary). It then returns the integer number, if the first character cannot be converted into a number, NaN will be returned ...
What is the most efficient way to convert a string to a number?
What would be the most efficient and safe method to convert a Decimal Integer String to a number in a time-critical loop or function where there are thousands (sometimes 100's thousands) of varying numbers strings (but are all Integers). I know of …