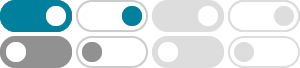
Java How To Convert a String to an Array - W3Schools
Convert a String to an Array. There are many ways to convert a string to an array. The simplest way is to use the toCharArray() method:
string to string array conversion in java - Stack Overflow
String str = "string to string array conversion in java"; String delimiter = " "; String strArray[] = str.split(delimiter); This creates the following array: // [string, to, string, array, conversion, in, java]
Get an array of Strings matching a pattern from a String
Jan 17, 2014 · String REGEX = "(?<=#)[A-Za-z0-9-_]+"; List<String> list = new ArrayList<String>(); Pattern pattern = Pattern.compile(REGEX); Matcher matcher = pattern.matcher(val); while(matcher.find()){ list.add(matcher.group()); }
Convert string to array in Java? - Stack Overflow
Mar 9, 2011 · Basically you would use Java's split() function: String str = "Name:Glenn Occupation:Code_Monkey"; String[] temp = str.split(" "); String[] name = temp[0].split(":"); String[] occupation = temp[1].split(":"); The resultant values would be: name[0] - Name name[1] - Glenn occupation[0] - Occupation occupation[1] - Code_Monkey
Convert String to Array in Java - Java2Blog
Dec 12, 2021 · In this tutorial, we have learned how to convert a String to an array by implementing five solutions which include using the toArray() method of Set, using StringTokenizor class, using the split() method of StringUtils class, using the split() method of String class, and concluded with using split() method of Pattern class.
String to Array in Java – How to Convert Strings to Arrays
Jul 6, 2023 · Converting a string to an array allows you to leverage array-specific operations like indexing, slicing, or joining to manipulate the data more efficiently or achieve specific formatting requirements.
How to Convert String to Array in Java | DigitalOcean
Aug 4, 2022 · String class split(String regex) can be used to convert String to array in java. If you are working with java regular expression , you can also use Pattern class split(String regex) method. Let’s see how to convert String to an array with a simple java class example.
Convert a String to Array in Java - Examples Java Code Geeks
Jan 19, 2021 · In this example, we are going to learn how to convert a string into an array in Java. We are going to discuss three different methods to break a string into an array. 1. Using substring () method. The string class provides substring () method to fetch part of a string.
Java: How to Convert a String to an Array - A Comprehensive …
Oct 27, 2023 · Learn multiple methods to convert a String to an Array in Java, including using the split() method, toCharArray(), and handling different scenarios with delimiters and data types. Includes detailed code examples and explanations for efficient string manipulation.
How to Convert String to Array in Java | devwithus.com
Jan 10, 2021 · The main purpose of this split() method is to break the passed string into an array according to a given pattern defined using the compile method. The following example will show you how to use the split() and the compile() methods to convert a String object to a String[] array using a regular expression: