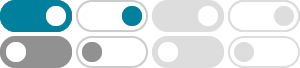
Convert image to binary using Python - GeeksforGeeks
Jan 3, 2023 · In this article, we are going to convert the image into its binary form. A binary image is a monochromatic image that consists of pixels that can have one of exactly two colors, usually black and white.
How to read the file and convert it to a binary image in Python
Feb 20, 2019 · I am new to Python, I want to read a image like jpg,png. and convert it to binary image. Here is my work: from PIL import Image import numpy def main( ): name= 'b.jpg' img= Image.open (name); for pixel in iter(img.getdata()): print(pixel) img.convert("1").show(); del image; if __name__=='__main__': main()
How can I read a binary file and turn the data into an image?
Basically what I want to do is take a file, bring its binary data (decimal of course) into an list and then generate a grayscale bitmap image using PIL based on that list.
Working with Binary Data in Python - GeeksforGeeks
Jun 22, 2020 · Convert image to binary using Python In this article, we are going to convert the image into its binary form. A binary image is a monochromatic image that consists of pixels that can have one of exactly two colors, usually black and white.
Python Tutorial: How to Perform Image Binarization in Python?
Oct 21, 2024 · Image binarization is a fundamental technique in image processing that can significantly enhance the analysis of images. By using Python and libraries like OpenCV, you can easily implement both simple and adaptive thresholding methods to …
Turning binary string into an image with PIL - Stack Overflow
If you have NumPy, you could instead use Image.fromarray: import Image import numpy as np value = "0110100001100101011011000110110001101111" carr = np.array([(255,255,255), (0,0,0)], dtype='uint8') data = carr[np.array(map(int, list(value)))].reshape(-1, 8, 3) img = Image.fromarray(data, 'RGB') img.save('/tmp/out.png', 'PNG')
5 Best Ways to Convert a Colored Image to a Binary Image Using …
Feb 27, 2024 · Given a standard colored image, we aim to transform it into a binary (black and white) image using different thresholding techniques available in the OpenCV library with Python. The most straightforward approach for converting a colored image to a …
Python Tutorial: How to Binarize Images in Python?
Oct 20, 2024 · In this tutorial, we will explore how to binarize images using Python, leveraging libraries like OpenCV and NumPy. Binarization simplifies the representation of an image by reducing the number of colors to just two. This is achieved by applying a threshold value to the grayscale image.
How to convert image to binary image - AiHints
In this article, you’ll see how to convert an image into a binary image using OpenCV in Python. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision. For converting an image into a binary image just follow these steps: Step 1: …
Convert RGB to Binary Image in Python (Black and White)
The complete and final Python code to convert an RGB or colored image into the binary is given below: import cv2 img = cv2.imread('imgs/mypic.jpg',2) ret, bw_img = cv2.threshold(img,127,255,cv2.THRESH_BINARY) cv2.imshow("Binary Image",bw_img) cv2.waitKey(0) cv2.destroyAllWindows()
- Some results have been removed