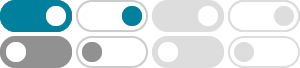
Is there a way to clear your printed text in python?
"Use this function to delete the last line in the STDOUT" #cursor up one line. sys.stdout.write('\x1b[1A') #delete last line. sys.stdout.write('\x1b[2K') print("hello") print("this line will be delete after 2 seconds") time.sleep(2) delete_last_line() Or os.system('clear') on …
How to clear only last one line in python output console?
to clear the line you are on use this: print("\033[K", end="\r") If you want to clear the previous line use both. If you only want to clear the line you are on (something used a lot in progress bars) use only the second one.
How to clear screen in python? - GeeksforGeeks
Aug 12, 2024 · Unfortunately, there’s no built-in keyword or function/method to clear the screen. So, we do it on our own. You can simply “cls” to clear the screen in windows. You can also only “import os” instead of “from os import system” but with that, you have to change system (‘clear’) to os.system(‘clear’).
Clearing previous terminal output in python - Stack Overflow
Apr 6, 2014 · Depending on whether you are on Windows or Linux, you can do: Example: print 'Hello...' print "All cleared!" Find the answer to your question by asking. See similar questions with these tags. Is there any way to clear the terminal's previous output? If so, how? Here is a code example to work off of. print "Hello..."
Python clear output - EyeHunts
Sep 14, 2023 · To clear the output of a specific cell, you can go to the “Cell” menu and select “Current Outputs” -> “Clear.” To clear the output of all cells, you can select “Edit” -> “Clear All Outputs.”
5 Best Ways to Clear Screen Using Python - Finxter
Feb 28, 2024 · Clearing the screen can be achieved by printing an escape sequence that instructs the terminal to clear its display. Here’s an example: Output: The screen will be cleared. No visible output will be observed.
how do you delete previous outputs so you can replace them ... - Reddit
Mar 2, 2022 · If you're asking how to completely clear the console each time you print, you can use the os.system () method which executes a command-line command. os.system('cls' if os.name == 'nt' else 'clear') or if you prefer to use an anonymous function: The command to clear the console differs depending on the operating system.
Top 7 Ways to Overwrite the Previous Output in Python
Nov 6, 2024 · Overwrite Previous Output in Python: Practical Approaches; Method 1: Basic Overwrite with Carriage Return; Method 2: Clearing the Line with ANSI Escape Codes; Method 3: Using sys.stdout.write() Method 4: Dynamic Message Length Handling; Method 5: Using Spaces to Clear Old Output; Method 6: Overwriting Multiple Lines; Method 7: Creating a Class ...
Python Overwrite Output Line - KyleTK
Sep 17, 2022 · Overwriting a line of output in a command line application in Python is a trivial task by just specifying a different ending in the print statement but with some additional technique is very versatile. Below I have a base script we will use to build off of. if __name__ == '__main__': for x in range (0, 101): print (f'Percent Complete: {x}%')
How to delete the last printed line in Python language
The cleanest approach, if possible, is to use \r, which goes back to the beginning of the line. Your next print will simply start from where the previous one started. For example: ... which is obviously not what we want. Whereas with \r: ... which is much better. If …