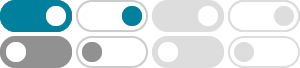
Java Program to Extract Digits from A Given Integer
Feb 24, 2022 · Given an integer in Java, your task is to write a Java program to convert this given integer into a binary number. Example: Input: = 45 Output: = 101101 Input: = 32 Output: = 100000 Integers: Integers are numbers whose base value is 10.
How to read each individual digit of a number in Java
Oct 4, 2016 · int num = number; //Set num equal to number as reference. //reads each digit of the scanned number and individually adds them together. //as it goes through the digits, keep dividing by 10 until its 0. while (num > 0) { int lastDigit = num % 10; . …
java - How to get the separate digits of an int number ... - Stack Overflow
Aug 2, 2010 · How can I get it in Java? To do this, you will use the % (mod) operator. print( number % 10); number = number / 10; The mod operator will give you the remainder of doing int division on a number. So, Because: Note: As Paul noted, …
java - Iterate through each digit in a number - Stack Overflow
Finding a happy number requires each digit in the number to be squared, and the result of each digit's square to be added together. In Python, you could use something like this: SQUARE[d] for d in str(n)
Extract Digits from a Given Integer in Java - Online Tutorials Library
For each character, we check if it is a digit using Character.isDigit(). If it is a digit, we convert it back to an integer using Character.getNumericValue() and store it in the digit variable. Finally, we display each digit using System.out.println().
Iterate Through Digits of a Number Java - Know Program
Iterate Through Digits of a Number Java Using Math.pow() & Operators. Let us see an another program to iterate through digits of a number Java from left to right direction using Math.pow() method, division and modulo operators. The Math.pow() method returns the double value therefore we have to convert them into int value using typecasting.
Java Program to Extract Digits from a Given Number
This is a Java Program to Extract Digits from A Given Integer. Enter any integer as input. After that we perform several operations like modulus and division to know the number of digits in given integer and then print each of the digits of the given number. Here is the source code of the Java Program to Extract Digits from A Given Integer.
How to Split an Integer Number Into Digits in Java | Baeldung
Jan 8, 2024 · When we work with integer numbers in Java, sometimes we need to break them into individual digits for various calculations or data manipulation tasks. In this tutorial, we’ll explore various approaches to splitting an integer number into its constituent digits using Java.
Digit Extraction in Java - Tpoint Tech
Mar 30, 2025 · One of the most straightforward ways to extract digits from a number is by using modulo (%) and division (/) operations. In this example, we start with the number 12345. The while loop continues until number becomes zero. In each iteration, we use the modulo operation (number % 10) to extract the last digit.
java - Extracting each digit in an int - Stack Overflow
Oct 10, 2016 · You can use Integer.toString(int) and then address each digit by index. int x = 1234; char[] s = Integer.toString(x).toCharArray(); System.out.println(s[0]); System.out.println(s[1]); System.out.println(s[2]); System.out.println(s[3]); If you don't want to convert your string to an array you can use the .charAt(int n) method on the string.