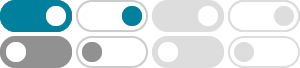
How to do a backspace in python - Stack Overflow
Dec 23, 2016 · Instead of your original for loop to print each number, try this: Explanation: [str(i) for i in range(1, times + 1)] is a list comprehension that returns a list of all your numbers, converted to strings so that we can print them. '+'.join(...) joins each element of your list, with a + in between each element. Alternatively:
python 3.x - deleting characters using backspace - Stack Overflow
Jul 8, 2021 · You should not modify the iterable inside its loop. I was approached this problem within 3 steps: Merged text and keys first; Count and collect index of backspace chars and effected normal chars; Deleted both of them in the merged object in reversed order; Try this out:
python - How to apply backspaces to a string? - Stack Overflow
You can apply backspaces to a string using regular expressions: while True: # if you find a character followed by a backspace, remove both. t = re.sub('.\b', '', s, count=1) if len(s) == len(t): # now remove any backspaces from beginning of string. return re.sub('\b+', '', t) s = t. Now:
Python While Loops - W3Schools
Python has two primitive loop commands: With the while loop we can execute a set of statements as long as a condition is true. Note: remember to increment i, or else the loop will continue forever. The while loop requires relevant variables to be ready, in this example we need to define an indexing variable, i, which we set to 1.
Examples, Usage, and Best Practices of Backspace (\b) in Python
Learn how to use the Backspace character (\b) in Python for string manipulation and formatting. Enhance your coding skills with practical examples!
Python while loop (infinite loop, break, continue, and more)
Aug 18, 2023 · Basic syntax of while loops in Python; Break a while loop: break; Continue to the next iteration: continue; Execute code after normal termination: else; Infinite loop with while statement: while True: Stop the infinite loop using keyboard interrupt (ctrl + c) Forced termination
8 Python while Loop Examples for Beginners - LearnPython.com
Feb 5, 2024 · In this article, we will examine 8 examples to help you obtain a comprehensive understanding of while loops in Python. Example 1: Basic Python While Loop. Let’s go over a simple Python while loop example to understand its structure and functionality: >>> i = 0 >>> while i . 5: >>> print(i) >>> i += 1 Result: 0 1 2 3 4
Python while Loops: Repeating Tasks Conditionally
In this tutorial, you'll learn about indefinite iteration using the Python while loop. You'll be able to construct basic and complex while loops, interrupt loop execution with break and continue, use the else clause with a while loop, and deal with infinite loops.
Python while loop examples for multiple scenarios
Dec 31, 2023 · In this example we will write a code to repeat python while loop a certain pre-defined number of times. The general syntax to achieve this would be: # do something here . i += 1. It is important that in such condition we increment the count in …
Backspace (\b) in Python : Usage, Examples, and Best Practices
How to Use Backspace (\b) in Python. The backspace character, represented as \b, is a control character that moves the cursor back one position in the output stream. In Python, it can be used in strings to create effects such as overwriting characters in terminal output.