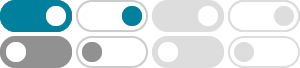
4 Ways to Find a Word in a List in Python: A Comprehensive Guide
Feb 15, 2023 · In Python, finding a specific word or element within a list is a familiar task developers often search for. This tutorial will teach us four effective methods to find a word in a list. We can use the in keyword to find a word in a list.
Python: how to determine if a list of words exist in a string
Feb 12, 2014 · word_list = ['word', 'foo', 'bar'] a_string = 'A very pleasant word to you.' for word in words_in_string(word_list, a_string): print word word EDIT: adaptation to use with a large file: Thanks to Peter Gibson for finding this the second fastest approach.
Filtering a list of strings based on contents - Stack Overflow
Jan 28, 2010 · This simple filtering can be achieved in many ways with Python. The best approach is to use "list comprehensions" as follows: >>> lst = ['a', 'ab', 'abc', 'bac'] >>> [k for k in lst if 'ab' in k] ['ab', 'abc'] Another way is to use the filter function. In Python 2: >>> filter(lambda k: 'ab' in k, lst) ['ab', 'abc']
Python check for word in list - Stack Overflow
Jul 16, 2013 · First, a word about data structures. Instead of lists, you should use sets, since you (apparently) only want a copy of each word. You can create sets out of your lists: input_words = set(word for line in separate for word in line) # since it is a list of lists correct_words = set(word_list) Then, it is simple as that:
Python | Extract Nth words in Strings List - GeeksforGeeks
Mar 18, 2024 · Using the re.findall() function to extract words from each string in the list and then selects the Nth word from each list if it exists. The result is a list containing the Nth words from each string.
Iterate over a list in Python - GeeksforGeeks
Jan 2, 2025 · Python provides several ways to iterate over list. The simplest and the most common way to iterate over a list is to use a for loop . This method allows us to access each element in the list directly.
Python Lists - GeeksforGeeks
Mar 11, 2025 · Python list slicing is fundamental concept that let us easily access specific elements in a list. In this article, we’ll learn the syntax and how to use both positive and negative indexing for slicing with examples.
Python Lists - W3Schools
Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets: Create a List: List items are ordered, changeable, and allow duplicate values.
How to search list of words for a given list of letters using Python
letters = {'t', 'u', 'v', 'w', 'x', 'y', 'z'} # a set, not a list for word in words: if letters.issuperset(word): print(word) The set.issuperset() method returns true if all elements of the iterable argument are in the set.
Lists in Python – A Comprehensive Guide - freeCodeCamp.org
Jun 3, 2021 · Python also has a built-in data structure called List that’s very similar to your shopping list. This post is a beginner-friendly tutorial on Python lists. Over the next few minutes, we'll get to know lists and cover some of the most common operations such as slicing through lists and modifying them using list methods.