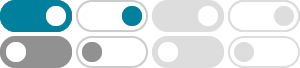
How would I check a string for a certain letter in Python?
Aug 25, 2024 · in keyword allows you to loop over a collection and check if there is a member in the collection that is equal to the element. In this case string is nothing but a list of characters: dog = "xdasds" if "x" in dog: print "Yes!" You can check a substring too: >>> 'x' in "xdasds" True >>> 'xd' in "xdasds" True >>> >>> >>> 'xa' in "xdasds" False
Python String find() Method - W3Schools
The find() method finds the first occurrence of the specified value. The find() method returns -1 if the value is not found. The find() method is almost the same as the index() method, the only difference is that the index() method raises an exception if the …
Finding values in a string using for loops in Python 3
Jan 30, 2017 · An easier method is to use a list comprehension: positions=[x for x in range(len(str1.split()))if str1.split()[x]==str2] This will contain all the indexes of str2 in str1.split() .
Check if String Contains Substring in Python - GeeksforGeeks
Jun 20, 2024 · Python uses many methods to check a string containing a substring like, find (), index (), count (), etc. The most efficient and fast method is by using an “in” operator which is used as a comparison operator. Here we will cover different approaches: Using __contains__” magic class. Check Python Substring in String using the If-Else.
python - Finding letters in a string - Stack Overflow
May 17, 2015 · Splitting a string returns a list of substrings. For example: "abc def ghi".split(" ") returns ["abc", "def", "ghi"]. You needn't split the string for what you're trying. Just loop over the string itself. string = "&*&)*&GOKJHOHGOUIGougyY^&*^x".lower() for char in string: if char.isalpha(): print(char)
Iterate over characters of a string in Python - GeeksforGeeks
Oct 27, 2024 · The simplest way to iterate over the characters in a string is by using a for loop. This method is efficient and easy to understand. Explanation: for char in s: This line loops through the string s, with char representing each character in …
5 Efficient Techniques to Check if a String Contains a Letter in Python
Feb 14, 2024 · Method 1: Using the “in” Operator. The “in” operator in Python is a straightforward and idiomatic way to check for the presence of a substring within a string. This method is highly readable and has the advantage of being easy to write and understand.
Python String find() Method - GeeksforGeeks
Oct 27, 2024 · The find() method in Python returns the index of the first occurrence of a substring within a given string. If the substring is not found, it returns -1 . This method is case-sensitive , which means “ abc ” is treated differently from “ ABC “.
Python String find (): How to Find a Substring in a String …
The find() is a string method that finds a substring in a string and returns the index of the substring. The following illustrates the syntax of the find() method: str .find ( sub [, start[, end] ]) Code language: CSS ( css )
Python String find() Method with Examples
Jan 24, 2024 · The find() method in Python is a built-in string method used to locate the index of the first occurrence of a specified substring within a string. It returns the index of the first character of the first occurrence of the substring, or -1 if the substring is not found.