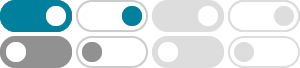
Python For Loops - W3Schools
With the for loop we can execute a set of statements, once for each item in a list, tuple, set etc. Print each fruit in a fruit list: The for loop does not require an indexing variable to set beforehand. Even strings are iterable objects, they contain a sequence of characters: Loop through the letters in the word "banana":
Python For Loop - Syntax, Examples
Python For Loop can be used to iterate a set of statements once for each item of a sequence or collection. The sequence or collection could be Range, List, Tuple, Dictionary, Set or a String. In this tutorial, we will learn how to implement for loop for each of the above said collections.
Python for Loop (With Examples) - Programiz
In Python, we use a for loop to iterate over sequences such as lists, strings, dictionaries, etc. For example, # access elements of the list one by one for lang in languages: print(lang) Output. In the above example, we have created a list named languages. Since the list has three elements, the loop iterates 3 times. The value of lang is.
Python For Loops - GeeksforGeeks
Dec 10, 2024 · In Python, enumerate () function is used with the for loop to iterate over an iterable while also keeping track of index of each item. This code uses nested for loops to iterate over two ranges of numbers (1 to 3 inclusive) and prints the value of i and j …
21 Python for Loop Exercises and Examples - Pythonista Planet
In Python programming, we use for loops to repeat some code a certain number of times. It allows us to execute a statement or a group of statements multiple times by reducing the burden of writing several lines of code. To get a clear idea about how a for loop works, I have provided 21 examples of using for loop in Python.
Loops in Python with Examples
In this article, we will learn different types of loops in Python and discuss each of them in detail with examples. So let us begin. In programming, the loops are the constructs that repeatedly execute a piece of code based on the conditions.
Loops in Python – For, While and Nested Loops - GeeksforGeeks
Mar 8, 2025 · Let us learn how to use for loops in Python for sequential traversals with examples. Explanation: This code prints the numbers from 0 to 3 (inclusive) using a for loop that iterates over a range from 0 to n-1 (where n = 4). We can use for loop to iterate lists, tuples, strings and dictionaries in Python.
python - Adding Numbers in a Range with for () Loop - Stack Overflow
for i in range(0,num+1) sum=sum+i. return sum. def run (n): total = 0 for item in range (n): total = total + item return total.
Python For Loop Example – How to Write Loops in Python
Apr 26, 2022 · But you can get around this by using the range() function to specify that you want to iterate through the numbers between two certain numbers. The range () function accepts two arguments, so you can loop through the numbers within the two arguments.
Python for Loops: The Pythonic Way – Real Python
Python’s for loop allows you to iterate over the items in a collection, such as lists, tuples, strings, and dictionaries. The for loop syntax declares a loop variable that takes each item from the collection in each iteration. This loop is ideal for repeatedly executing a block of code on each item in the collection.