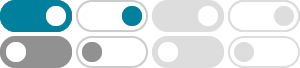
Check Palindrome (for numbers) - Algorithm, flowchart, …
Given a number, write a algorithm, flowchart, pseudocode to check if it is palindrome or not. A string is said to be palindrome if reverse of the string is same as string. For example, 1221 is palindrome, but 1223 is not palindrome.
Creating a Flowchart for Python Palindromic Pattern Generation: …
Creating palindromic patterns in Python requires a systematic approach to generate symmetrical number sequences. This flowchart breaks down the process into logical steps, making it easier to understand and implement the pattern generation algorithm. Let's explore how each component works together to create beautiful palindromic patterns.
Algorithm and Flowchart to check whether a string is Palindrome …
Oct 16, 2022 · In simple words, a string is said to be palindrome when the string read backwards is equal to the given string or when both the string and its reverse are equal. Now let’s take a look at the algorithm and flowchart to check whether a given string is …
Graph visualisation basics with Python Part I: Flowcharts
Apr 18, 2022 · if string == reverse_string: print (f"{string} is a palindrome.") else: print (f"{string} is not a palindrome.") The code to get the flowchart for this problem using SchemDraw is given in the gist below.
Palindrome String Flowchart [ 2024 ] | TestingDocs.com
Palindrome String Flowchart. In this tutorial, we will design a Flowgorithm flowchart to determine if a string is a palindrome or not. A palindrome string is a string that is spelled identical forward or in the reverse. Some example palindrome strings are: adcda; madam; radar; Some examples of non-palindrome strings are:
Python Programs to Check Palindrome Number - PYnative
Apr 8, 2025 · Explanation. 1. Initialize the variables. number holds the value you want to check (in this case, 12321).; original_number is a copy of the number so you can compare it later after reversing it.; reversed_number starts at 0 and will be used to build the reversed version of the number.; 2. Use while loop to reverse the digits of the number. Extract the last digit using the modulus operation ...
Write an algorithm and draw a flow chart to check whether the …
Jan 6, 2025 · Code Implementation: Programming Language: Python. Code Content: def is_palindrome(number): """ Checks if a number is a palindrome. Args: number: An integer. Returns: True if the number is a palindrome, False otherwise.
Python Program to Check if a String is Palindrome or Not
Feb 21, 2025 · The task of checking if a string is a palindrome in Python involves determining whether a string reads the same forward as it does backward. For example, the string "madam" is a palindrome because it is identical when reversed, whereas "hello" is not.
Palindrome in Python - AskPython
May 6, 2021 · Let’s check for palindrome numbers using Python. n = input() n = int(n) copy_n=n result = 0 while(n!=0): digit = n%10 result = result*10 + digit n=int(n/10) print("Result is: ", result) if(result==copy_n): print("Palindrome!") else: print("Not a Palindrome!")
Write an algorithm and draw a corresponding flowchart to test
Oct 19, 2019 · Write an algorithm and draw a corresponding flowchart to test whether a given word is a palindrome. str = input () if str == str [::-1]: print (str, "is a palindrome.") else: print (str, "is not a palindrome") First line: asks for an input string. Second line onwards: checks if the original string is the same as the reverse of the string.
- Some results have been removed