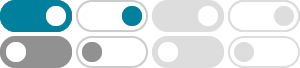
How to recognize a repeating pattern in an array in javascript
If I have an array like. const arr = [1, 5, 7, 5, 13, 8, 1, 7, 3, 8, 5, 2, 1, 5, 7]; What would be the best way of finding that the array starts to repeat itself? In this instance that the first three numbers and the last three numbers are in a repeating pattern.
Find highest recurring duplicates in a javascript array
Mar 19, 2017 · The standard solution to find duplicates (in O (n) time) is to walk linearly through the list and add each item to a set. Each time, before doing so, check if it is already in the set. If it is, you've found a duplicate. if (seen.has(item)) { console.log(item + " is a duplicate"); } …
What are all the ways to find repetitive elements in array in javascript
Dec 17, 2019 · I have been asked in the interview to find repetitive elements in array. I have found using for loop, but the interviewer asked for more improved way to find out, with effect to performance with out for loop .
JavaScript Program to Find Duplicate Elements in an Array
Apr 7, 2025 · In JavaScript, array methods such as filter () and indexOf () can be used to find duplicates in a concise way. filter () creates a new array containing elements that satisfy a condition. arr.indexOf (value) returns the first occurrence index of value.
Find duplicate or repeat elements in js array - DEV Community
Aug 10, 2020 · There are a couple of ways to count duplicate elements in a javascript array. by using the forEach or for loop. Iterate over the array using a for loop.
Find the Repeating & Missing Numbers in JavaScript Array
Mar 8, 2024 · JavaScript allows us to find the repeating and missing number in a given array which includes numbers from 1 to N range. We are given an array that has numbers present from 1 to N range, where N is any natural number. One number is present two times and one number is missing from the range.
How to find the mode (most repeating number) of an array in JavaScript
Jan 10, 2021 · To get our object to look like that, we introduce an if...else block. For each element in the array input, if the element is already a key in the object, we increment that key's value by one. If the element is not already in the object, we make …
How to recognize a repeating pattern in an array in javascript
To recognize a repeating pattern in an array using JavaScript, you can employ various techniques depending on the nature of the pattern you're looking for. Here's a general approach to get you started:
Find duplicates in an array using javaScript - Flexiple
Mar 14, 2022 · Learn how to write a JavaScript program to efficiently find duplicate elements in an array. Discover tips, tricks, and code examples for quick implementation.
How to find duplicates in an array using JavaScript - Atta-Ur …
Jul 3, 2021 · Learn how to check if an array contains duplicate values using indexOf(), set object, and iteration in JavaScript.