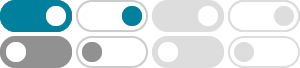
Java Program to Find Factorial of a Number Recursively
Feb 21, 2023 · In this article, we will learn how to write a program for the factorial of a number in Java. Formulae for Factorialn! = n * (n-1) * (n-2) * (n-3) * ........ * 1 Example: 6! == 6*5*4*3*2*1 = 720. 5! 4 min read
Java Program to Find Factorial of a Number Using Recursion
In this program, you'll learn to find and display the factorial of a number using a recursive function in Java.
Recursion in Java - GeeksforGeeks
Jul 12, 2024 · 1. Factorial Using Recursion. The classic example of recursion is the computation of the factorial of a number. The factorial of a number N is the product of all the numbers between 1 and N. The below-given code computes the factorial of the numbers: 3, 4, and 5. 3= 3 *2*1 (6) 4= 4*3*2*1 (24) 5= 5*4*3*2*1 (120) Below is the implementation of ...
Factorial using Recursion in Java - Stack Overflow
Nov 18, 2011 · First you should understand how factorial works. Lets take 4! as an example. 4! = 4 * 3 * 2 * 1 = 24 Let us simulate the code using the example above: int fact(int n) { int result; if(n==0 || n==1) return 1; result = fact(n-1) * n; return result; }
Java Program to Find Factorial Using Recursion - Java Guides
This blog post will demonstrate how to calculate the factorial of a number using recursion in Java, a fundamental concept in programming that involves a function calling itself. 2. Program Steps. 1. Define a recursive method to calculate the factorial. 2. Read the number for which the factorial is to be calculated from the user. 3.
Java Recursive Method: Calculate the factorial of a number
Mar 11, 2025 · Write a Java recursive method to calculate the factorial of a given positive integer. Sample Solution: Java Code: public static int calculateFactorial(int n) { // Base case: factorial of 0 is 1. if (n == 0) { return 1; // Recursive case: multiply n with factorial of (n-1) return n * calculateFactorial(n - 1);
How to Find Factorial of a Number in Java Using Recursion
Feb 21, 2024 · To find the factorial of a number in Java using recursion, create a recursive function that takes a number and multiplies it with its preceding numbers recursively until the base condition is fulfilled.
Factorial Using Recursion in Java — Step-by-Step Guide with Code Example
Here’s a simple yet effective code example that demonstrates how to calculate the factorial using recursion in Java: // Recursive method to calculate factorial. public static int...
How to find Factorial in Java using Recursion and Iteration - Example …
Without wasting any more of your time, let's jump into the two solutions we can use to calculate factorials in Java. In the first solution, we will use recursion, where a method calls itself for repetition, and in the second solution, we will use loops …
How to calculate Factorial in Java? Iteration and Recursion Example ...
Dec 10, 2022 · You can calculate factorial of a given number in Java program either by using recursion or loop. The factorial of a number is equal to number*fact(number-1), which means its a recursive...
- Some results have been removed