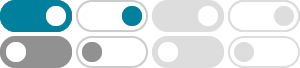
Queue in Python - GeeksforGeeks
Jul 9, 2024 · Python Queue can be implemented by the following ways: List is a Python’s built-in data structure that can be used as a queue. Instead of enqueue () and dequeue (), append () and pop () function is used.
Stack and Queues in Python - GeeksforGeeks
May 9, 2022 · Unlike C++ STL and Java Collections, Python does have specific classes/interfaces for Stack and Queue. Following are different ways to implement in Python. Stack works on the principle of “Last-in, first-out”. Also, the inbuilt functions in Python make the …
Enqueue in Queues in Python - GeeksforGeeks
Apr 16, 2024 · In this article, we will see the methods of Enqueuing (adding elements) in Python. Enqueue: The act of adding an element to the rear (back) of the queue. Dequeue: The act of removing an element from the front of the queue. FIFO (First-In-First-Out): Elements are processed in the order they are added.
Queue Class, dequeue and enqueue ? python - Stack Overflow
Dec 13, 2013 · If you want to test that your queue class works properly, you will need to write test functions, and then call them. For example: def test_queue(): q = Queue() for i in range(10): q.enqueue(i) for i in range(10): value = q.dequeue() if value != i: print('Value #{} should be {} but is {}'.format(i, i, value)) try: value = q.dequeue() except ...
Implement A Queue using Two Stacks Python - Stack Overflow
class CreatingQueueWithTwoStacks: def __init__(self): self.stack_1 = Stack() self.stack_2 = Stack() def enqueue(self, item): self.stack_1.push(item) def dequeue(self): if not self.stack_1.is_empty(): while self.stack_1.size() > 0: self.stack_2.push(self.stack_1.pop()) res = self.stack_2.pop() while self.stack_2.size() > 0: self.stack_1.push ...
Python Program to Implement Queues using Stack - Sanfoundry
This is a Python program to implement a queue using two stacks. The program creates a queue using stacks and allows the user to perform enqueue and dequeue operations on it. 1. Create …
Implementing Queues Using Stacks in Python: A Comprehensive …
Mar 7, 2024 · Using recursion, we can simulate a queue with a single stack for storage. The recursion stack implicitly becomes the second stack. During deQueue, if the storage stack has more than one element, we use recursion to store the popped item temporarily and push it back after we reach the base case.
Stacks and Queues using Python - 101 Computing
Mar 10, 2018 · Stacks and Queues are two key data structures often used in programming. A queue is a FIFO data structure: First-In First-Out in other words, it is used to implement a first come first served approach. An item that is added (enqueue) at the end of a queue will be the last one to be accessed.
Python Stacks, Queues, and Priority Queues in Practice
Adding an element to the FIFO queue is commonly referred to as an enqueue operation, while retrieving one from it is known as a dequeue operation. Don’t confuse a dequeue operation with the deque (double-ended queue) data type that you’ll learn about later!
Python Program to Implement Stack using Queue - Sanfoundry
1. Create a class Queue. 2. Define methods enqueue, dequeue, is_empty and get_size inside the class Queue. 3. Create a class Stack with instance variable q initialized to an empty queue. 4. Pushing is done by enqueuing data to the queue. 5. To pop, the queue is dequeued and enqueued with the dequeued element n – 1 times where n is the size of ...
- Some results have been removed