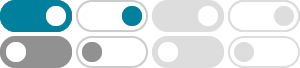
C Program to Implement Circular Queue - GeeksforGeeks
Jun 2, 2024 · The circular queue can be implemented using both an array or a circular linked list but it is most beneficial for array implementation as it resolves the stack overflow condition of the linear implementation even when the whole array is empty.
Circular Array Implementation of Queue - GeeksforGeeks
Mar 28, 2025 · In this article, we will discuss how to create a dynamic circular queue using a circular array having the following functionality: Front(): Get the front item from the queue.Back(): Get the last item from the queue.Push(X): Push the X in the queue at the end of the queue.Pop(): Delete an element fro
C Program to implement circular queue using arrays
Apr 10, 2017 · Here’s simple Program to implement circular queue using arrays in C Programming Language. What is Queue ? Queue is also an abstract data type or a linear data structure, in which the first element is inserted from one end called REAR , and the deletion of existing element takes place from the other end called as FRONT.
Circular Queue Data Structure - Programiz
Circular Queue works by the process of circular increment i.e. when we try to increment the pointer and we reach the end of the queue, we start from the beginning of the queue. Here, the circular increment is performed by modulo division with the queue size. That is, The circular queue work as follows: 1. Enqueue Operation. 2. Dequeue Operation.
Circular Queue Using Array in C - PrepInsta
In this post we will learn on how we can implement circular queue using array in C . Circular queues are extension of linear queues where the max size of queue is always available for insertion.
Problem with display function inside circular queue
Dec 7, 2021 · #include <stdio.h> # define MAX 3 int queue[MAX]; // array declaration int front=-1; int rear=-1; // function to insert an element in a circular queue void enqueue(int element) { if(front==-1 && rear==-1) // condition to check queue is empty { front=0; rear=0; queue[rear]=element; } else if((rear+1)%MAX==front) // condition to check queue is ...
How do I implement a circular list (ring buffer) in C?
Mar 4, 2016 · Use an array and keep a variable P with the first available position. Increase P every time you add a new element. To know the equivalent index of P in your array do (P % n) where n is the size of your array.
c++ - Circular queue Display - Stack Overflow
Aug 12, 2020 · In void Queue::displayQueue () { if (front == -1) { printf ("\nQueue is Empty"); return; } printf ("\nElements in Circular Queue are: "); if (rear >= front) { ...
Circular Queue Using Array in C - CS Taleem
But in this lecture will implements Circular Queue using array in C using dynamic memory allocation. Let’s break it down into its components. First look at circular queue behaviors through the following diagram. Global Variables. int *queue: A pointer for dynamically allocated memory for the queue. int front, rear: Indices to track the front ...
Circular Queue in C using Array - Code Revise
A circular queue is an abstract data type that includes a collection of data and allows for data addition at the end of the queue and data removal at the beginning. Circular queues have a fixed size. Circular queue follows FIFO( First In, First Out ) principle.
- Some results have been removed