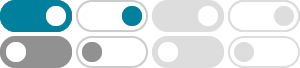
How can I remove a key from a Python dictionary?
To delete a key regardless of whether it is in the dictionary, use the two-argument form of dict.pop():. my_dict.pop('key', None)
python - Delete an element from a dictionary - Stack Overflow
May 1, 2011 · How do I delete an item from a dictionary in Python? Without modifying the original dictionary, how do I obtain another dictionary with the item removed? See also How can I remove a key from a Python
Delete a dictionary item if the key exists - Stack Overflow
Is there any other way to delete an item in a dictionary only if the given key exists, other than: if key in mydict: del mydict[key] The scenario is that I'm given a collection of keys to be removed from a given dictionary, but I am not certain if all of them exist in the dictionary. Just in case I miss a more efficient solution.
python - Removing multiple keys from a dictionary safely - Stack …
Jan 25, 2012 · Python Delete a key from a dictionary with multiple keys. 0. Remove keys but keep values from dictionary ...
Proper way to remove keys in dictionary with None values in Python
Nov 19, 2015 · Python3 recursive version. def drop_nones_inplace(d: dict) -> dict: """Recursively drop Nones in dict d in-place and return original dict""" dd = drop_nones(d) d.clear() d.update(dd) return d def drop_nones(d: dict) -> dict: """Recursively drop Nones in dict d and return a new dict""" dd = {} for k, v in d.items(): if isinstance(v, dict): …
python - Deleting items from a dictionary with a for loop - Stack …
May 11, 2014 · However, Python 3's dict.items returns a view object that reflects all changes made to the dictionary. This is the reason the solution above only works in Python 2. You may convert my_dict.items() to list (tuple etc.) to make it Python 3-compatible. Another way to approach the problem is to select keys you want to delete and then delete them
What is the best way to remove a dictionary item by value in …
Mar 24, 2015 · (See for instance get key by value in dictionary.) Delete this dict element or elements (based on the found keys) from myDict (For more information, see Delete an element from a dictionary.) So, what do you think now is the most elegant and most “pythonic” way to implement this problem in Python?
python - Remove dictionary from list - Stack Overflow
Edit: as some doubts have been expressed in a comment about the performance of this code (some based on misunderstanding Python's performance characteristics, some on assuming beyond the given specs that there is exactly one dict in the list with a value of 2 for key 'id'), I wish to offer reassurance on this point.
How to delete items from a dictionary while iterating over it?
Mar 22, 2011 · You can use .keys() et al for this (in Python 3, pass the resulting iterator to list). Could be highly wasteful space-wise though. Iterate over mydict as usual, saving the keys to delete in a seperate collection to_delete. When you're done iterating mydict, delete all items in to_delete from mydict. Saves some (depending on how many keys are ...
Elegant way to remove fields from nested dictionaries
Aug 4, 2010 · tbh I think your fisrt version was better, not returning the dictionary because as you said the original will already have the updated keys and you are not "wasting" the return value to return something already existent and the method could be modified in the future to return for example the number of values removed without changes to the already existent calling code.