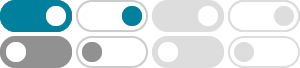
Initialize a string variable in Python: "" or None?
If you just want a default value, "" is probably better, because its actually a string, and you can call string methods on it. If you went with None, these would lead to exceptions. If you wish to indicate to future maintainers that a string is required here, "" can help with that. Complete side note: If you have a loop, say:
Python Variable Declaration - Stack Overflow
Jun 13, 2012 · In Python, strings are immutable. They cannot be modified. When you do: a = 'hi ' b = a a += 'mom' You do not change the original 'hi ' string. That is impossible in Python. Instead, you create a new string 'hi mom', and cause a to stop being a name for 'hi ', and start being a name for 'hi mom' instead.
What is the best way to create a string array in python?
The best and most convenient method for creating a string array in python is with the help of NumPy library. Example : import numpy as np arr = np.chararray((rows, columns))
How to write string literals in Python without having to escape …
If you use a raw string then you still have to work around a terminal "\" and with any string solution you'll have to worry about the closing ", ', ''' or """ if it is included in your data. That is, there's no way to have the string ' ''' """ " \ properly stored in any Python string literal without internal escaping of some sort.
string - Declaring encoding in Python - Stack Overflow
Sep 3, 2012 · @imrek then instead of declaring an encoding of UTF-8, declare the encoding that your file actually uses. It has nothing to do with the Python version and everything to do with the file itself. – Karl Knechtel
How do I create a multiline Python string with inline variables?
Apr 12, 2012 · Alternatively, you can also create a multiline f-string with triple quotes where literal newlines are considered part of the string. However, any spaces at the start of the line would also be part of the string, so it can mess with code indentation. multiline_string = f"""I will {string1} there. I will go {string2}. {string3}."""
Explicitly declaring a variable type in Python - Stack Overflow
Python has no type declarations. Python 3 introduces something called function annotations, which Guido sometimes refers to as "the thing that isn't type declarations," because the most obvious use of it will be to provide type information as a hint. As others have mentioned, various IDEs do a better or worse job at auto-completing.
String-based enum in Python - Stack Overflow
Oct 29, 2019 · If associated string values are valid Python names then you can get names of enum members using .name property like this: from enum import Enum class MyEnum(Enum): state1=0 state2=1 print (MyEnum.state1.name) # 'state1' a = MyEnum.state1 print(a.name) # 'state1' If associated string values are arbitrary strings then you can do this:
python - How to define a two-dimensional array? - Stack Overflow
Jul 12, 2011 · In Python you will be creating a list of lists. You do not have to declare the dimensions ahead of time, but you can. For example: matrix = [] matrix.append([]) matrix.append([]) matrix[0].append(2) matrix[1].append(3) Now matrix[0][0] == 2 and matrix[1][0] == 3. You can also use the list comprehension syntax.
Initialising an array of fixed size in Python - Stack Overflow
The question specified ".. yet to be populated with values". The Python docs indicate "None is typically used to represent absence of a value". My example produced such a list of the defined size, in the shortest amount of code. Its the closest thing in idiomatic Python at the time the question was asked. –